Learn About VueJS and How to Use It in Frontend Development
- Published on
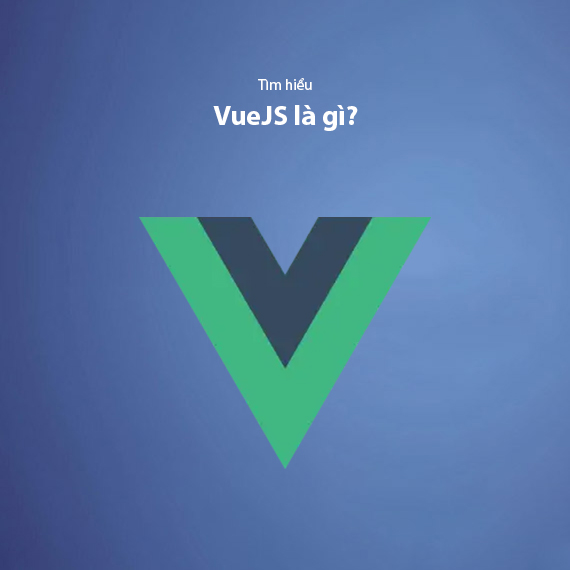
- What is VueJS?
- How VueJS Works
- Basic Structure of a VueJS Project
- Main Directory
- Other Directories
- Single File Components (SFC)
- Advantages and Disadvantages of VueJS
- Advantages of VueJS
- Disadvantages of VueJS
- Summary Table
- Guidelines for Effectively Using VueJS in Frontend Development
- Start with Basic Components
- Effective State Management
- Optimizing Structure and Performance
- Improving SEO with Server-Side Rendering (SSR)
- How to Optimize Performance in VueJS
- Utilize Lazy Loading for Components and Pages
- Use v-once for Non-Dynamic Elements
- Avoid Unnecessary v-for
- Use Computed Properties Instead of Methods
- Optimize CSS with scoped and CSS-in-JS
- Choose Lightweight Libraries and Plugins
- Frequently Asked Questions About VueJS
- Is VueJS Suitable for Beginners?
- Can VueJS Be Used for Large Projects?
- Does VueJS Support SEO?
- How Does VueJS Compare to Other Frameworks?
- Are There Any Disadvantages of VueJS?
- Does VueJS Support Mobile Applications?
- Conclusion
What is VueJS?
Vue.js (commonly referred to as Vue) is an open-source JavaScript framework used to build user interfaces (UI) and develop web applications. Introduced in 2014 by Evan You, Vue.js quickly gained popularity in the development community due to its flexibility and ease of use. One of Vue's standout features is its ability to seamlessly integrate with existing projects or work alongside other libraries. Additionally, Vue supports building Single Page Applications (SPA) with clear structure and robust scalability.
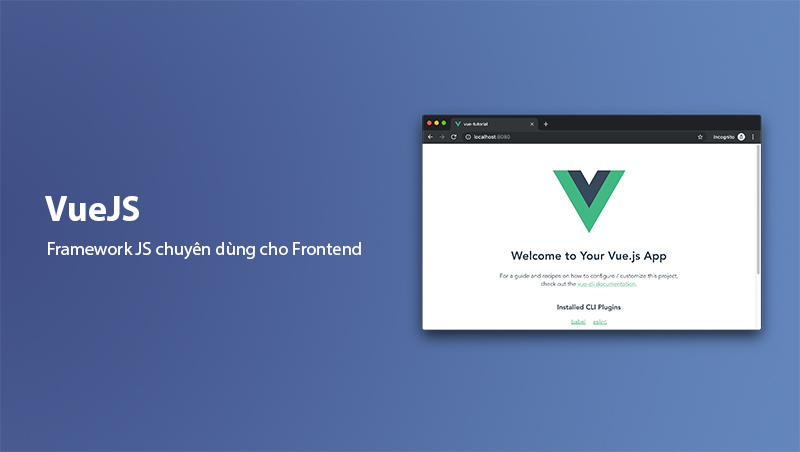
Vue.js primarily focuses on the view layer, optimizing the user experience without demanding excessive resources. One of Vue's significant advantages is its straightforward syntax, which is suitable for both beginners and seasoned developers. The Vue.js community also offers a rich ecosystem of tools, plugins, and libraries like Vue Router and Vuex, enhancing the framework's power and convenience for application development.
How VueJS Works
Vue.js operates on a reactivity system, where the data and interface remain automatically synchronized. When a piece of data in the application changes, Vue detects the modification through an observer mechanism and automatically updates the relevant UI components without requiring manual intervention. This feature makes building applications easier and more efficient, especially for complex projects.
A crucial aspect of Vue is the Virtual DOM. When data changes, Vue does not immediately update the Real DOM. Instead, it creates a Virtual DOM copy to analyze the differences (diffing). Then, only the affected elements are updated in the Real DOM, significantly improving the application's performance, especially for data-heavy or highly interactive web pages.
Moreover, Vue.js enables developers to use Directives (such as v-bind
, v-if
, v-for
) to declaratively define rendering rules for the interface. This mechanism reduces repetitive code and optimizes the development process. Single File Components (SFC) is another powerful feature, allowing developers to encapsulate HTML, CSS, and JavaScript in a single .vue
file for easier management and code reuse.
Basic Structure of a VueJS Project
A typical Vue.js project has a clear directory structure designed for easy management and scalability. Below is the common structure when using Vue CLI – the official Vue project creation tool:
Main Directory
src/
: The most important directory containing the project's source code. Key components include:main.js
: The application's entry point where Vue is initialized and linked to the main HTML file.App.vue
: The root component of the application, housing all the child components.components/
: A directory for reusable UI components, such as buttons and dialogs.assets/
: Stores static resources like images, CSS files, or fonts.router/
(if applicable): Contains Vue Router configuration for managing page navigation.store/
(if applicable): A directory for using Vuex to manage global state.
Other Directories
public/
: Contains static HTML files, such asindex.html
, where Vue injects its components.node_modules/
: Automatically generated by npm/yarn, housing the project's dependencies.package.json
: Defines the project's metadata, dependencies, and scripts.
Single File Components (SFC)
The .vue
file is a hallmark of Vue.js, encapsulating HTML, CSS, and JavaScript in a single file. A typical .vue
file structure includes three sections:
<template>
<div>
<h1>Welcome to Vue!</h1>
</div>
</template>
<script>
export default {
name: 'WelcomeComponent',
};
</script>
<style>
h1 {
color: blue;
}
</style>
Advantages and Disadvantages of VueJS
Advantages of VueJS
-
Simple and Easy-to-Learn Syntax VueJS has clear, understandable syntax, making it easy for developers, even beginners, to get started and build applications. With detailed and rich documentation, Vue is an ideal choice for diving into modern frontend frameworks.
-
Flexible Integration Vue can be integrated into existing projects without requiring significant structural changes. You can use Vue for a small part of your UI or build an entire application with the framework.
-
High Performance Vue leverages Virtual DOM, minimizing updates and improving rendering speed. This makes Vue highly efficient for both small and complex applications.
-
Robust Ecosystem Vue provides powerful tools like Vue CLI, Vue Router (for navigation management), and Vuex (for state management), enabling seamless and efficient frontend development.
-
Active Community Support Although smaller than React or Angular communities, the Vue.js community is rapidly growing, offering numerous libraries, plugins, and helpful resources.
Disadvantages of VueJS
-
Challenges in Large-Scale Projects Vue is not as commonly used in large enterprise projects as React or Angular, making it harder to find resources or expert-level support for complex applications.
-
Decentralized Ecosystem Unlike Angular, Vue does not have centralized management for plugins and tools. Many of its plugins or libraries are third-party, which can complicate synchronization and selection.
-
Limited Recognition in Large Enterprises While popular among startups and small projects, Vue has not been widely adopted by large corporations, which may limit job opportunities related to the framework.
Summary Table
Advantages | Disadvantages |
---|---|
|
|
Pro Tip: If you're deciding on a framework for a new project, check out the article Detailed Comparison of the Best Frontend Frameworks of 2024 for guidance.
Guidelines for Effectively Using VueJS in Frontend Development
Start with Basic Components
Leverage Single File Components (SFC) to create independent, reusable UI components. This practice ensures your application is easier to maintain as the project grows. Each .vue
file should be clearly organized into the following sections:
- Template: Defines the HTML to be displayed.
- Script: Contains the logic and data.
- Style: Handles the component's design.
Example:
<template>
<button @click="handleClick">Click Me</button>
</template>
<script>
export default {
methods: {
handleClick() {
alert('Welcome to VueJS!');
},
},
};
</script>
<style scoped>
button {
background-color: #42b983;
color: white;
border: none;
padding: 10px 20px;
cursor: pointer;
}
</style>
Effective State Management
In large applications, managing state becomes increasingly complex. Use Vuex, VueJS's official state management library, to store and share data between components easily.
Examples:
- Use Vuex to manage a shopping cart in an e-commerce application.
- Manage login status or user data efficiently.
Optimizing Structure and Performance
- Code splitting: Use Vue Router's lazy loading feature to load components or pages only when needed, reducing initial load times.
- Debounce and Throttle: For events like input or scrolling, apply these techniques to reduce the number of processes triggered.
- Use computed properties: Instead of handling logic directly in templates, utilize computed properties to ensure the UI updates automatically and efficiently.
Improving SEO with Server-Side Rendering (SSR)
Use Nuxt.js, a framework built on Vue, to support SSR, improving SEO for VueJS applications. This is particularly important for pages that need to rank well on search engines.
How to Optimize Performance in VueJS
To ensure your VueJS application runs quickly and stably, performance optimization is essential. Here are the most effective ways to improve VueJS app performance:
Utilize Lazy Loading for Components and Pages
Lazy loading reduces page load times by loading components or modules only when needed. This is especially useful for applications with multiple pages or components that aren't always displayed. Use dynamic import like this:
const LazyComponent = () => import('./components/LazyComponent.vue');
For Vue Router, you can also apply lazy loading to routes:
const routes = [
{
path: '/about',
component: () => import('./views/AboutView.vue'),
},
];
v-once
for Non-Dynamic Elements
Use For elements in the UI that do not need to update, use the v-once
directive to render them only once:
<h1 v-once>This is a static heading</h1>
This reduces unnecessary re-renders and enhances performance.
v-for
Avoid Unnecessary v-for
loops can become resource-intensive when applied to large lists. Always pair them with key
to help Vue identify and manage elements efficiently:
<ul>
<li v-for="(item, index) in items" :key="index">{{ item }}</li>
</ul>
Use Computed Properties Instead of Methods
Computed properties are recalculated only when their dependencies change, unlike methods which are re-executed on every render. This reduces application load:
computed: {
fullName() {
return `${this.firstName} ${this.lastName}`;
},
}
scoped
and CSS-in-JS
Optimize CSS with In Vue, limiting CSS to specific components helps reduce conflicts and improves UI processing speed. Use the scoped
attribute in <style>
:
<style scoped>
.button {
background-color: #42b983;
}
</style>
Choose Lightweight Libraries and Plugins
Instead of integrating heavy libraries, look for lighter alternatives, such as:
- Using Axios instead of other bulkier HTTP libraries.
- Only use essential Vue plugins, avoiding unnecessary modules.
Frequently Asked Questions About VueJS
Below are some common questions about VueJS, especially from beginner developers:
Is VueJS Suitable for Beginners?
Yes. VueJS is designed with a straightforward, clear syntax, and its official documentation is very detailed. This makes it easy for beginners to get started and develop applications without needing advanced JavaScript knowledge. Compared to frameworks like React or Angular, Vue has a gentler learning curve.
Can VueJS Be Used for Large Projects?
Yes, but careful planning and architecture are required. For large projects, use tools like Vuex for state management, Vue Router for navigation, and consider TypeScript to maintain clean, scalable code. However, for very large systems, React or Angular are still preferred by large enterprises due to their broader ecosystems and enterprise-level support.
Does VueJS Support SEO?
VueJS, when used as a SPA, has limitations with SEO because content is loaded via JavaScript. However, this can be addressed with Server-Side Rendering (SSR) or frameworks like Nuxt.js, significantly improving SEO by pre-rendering content on the server.
How Does VueJS Compare to Other Frameworks?
- VueJS vs React: Vue has simpler syntax, while React is more powerful due to extensive enterprise community support. Vue uses a template-based approach, whereas React uses JSX.
- VueJS vs Angular: Vue is lighter and easier to learn than Angular. Angular is a monolithic framework with many built-in features, while Vue focuses on flexibility and scalability.
Are There Any Disadvantages of VueJS?
Some disadvantages of VueJS include:
- Limited resources compared to React or Angular.
- Less common in large enterprise projects.
- Some libraries are not officially developed and rely on third-party developers.
Learn More: If you want to compare VueJS with React, read the article What is React? A Guide to Using React in Frontend Development.
Does VueJS Support Mobile Applications?
VueJS can be used for mobile application development through frameworks like NativeScript Vue or Quasar Framework, which help create cross-platform apps based on Vue.
Conclusion
VueJS is a powerful frontend framework that is beginner-friendly and well-suited for seasoned web developers. With its simple syntax, flexible integration, and high performance, VueJS is an excellent choice for small to medium-sized projects. Despite some limitations for large enterprise applications, Vue proves its worth through its rich ecosystem and active community support.
If you're looking for a tool to build modern user interfaces or develop Single Page Applications (SPA), VueJS will undoubtedly meet your expectations. Tools like Vue CLI, Vue Router, and Vuex allow you to create robust and optimized applications quickly.
Remember, choosing a framework depends not only on its features but also on the project's scale and specific requirements. If you're interested in performance optimization or improving SEO, consider solutions like Nuxt.js or adopting the recommended optimization techniques.
Latest Posts
What is API Monitoring? A Guide to Effective API Management
Discover API Monitoring, how to effectively monitor APIs, and its crucial role in optimizing performance and ensuring system stability.
What Is an API? Basic Knowledge About Application Programming Interface
Learn about APIs, how they work, and their critical role in connecting and integrating software systems today.
What Is API Gateway? Its Role in Microservices Architecture
Learn about API Gateway, its critical role in Microservices architecture, and how it helps optimize management and connection of services within a system.
What is Cache? A Guide to Clearing Cache on All Major Browsers
Learn about cache, its benefits in speeding up website access, and how to clear cache on popular browsers.
Related Posts
What is API Monitoring? A Guide to Effective API Management
Discover API Monitoring, how to effectively monitor APIs, and its crucial role in optimizing performance and ensuring system stability.
What Is an API? Basic Knowledge About Application Programming Interface
Learn about APIs, how they work, and their critical role in connecting and integrating software systems today.
What Is API Gateway? Its Role in Microservices Architecture
Learn about API Gateway, its critical role in Microservices architecture, and how it helps optimize management and connection of services within a system.
What is Cache? A Guide to Clearing Cache on All Major Browsers
Learn about cache, its benefits in speeding up website access, and how to clear cache on popular browsers.
