Understanding ReactJS - The Core Foundation of Modern Web Development
- Published on
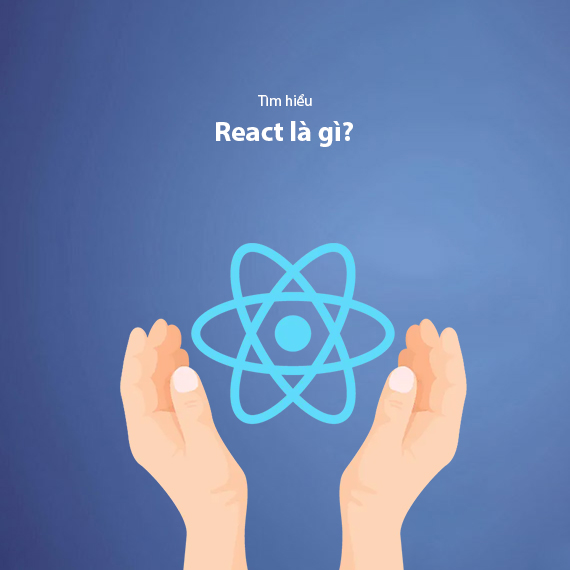
- Key Components in ReactJS
- Components
- JSX (JavaScript XML)
- Props (Properties)
- State
- Hooks
- Virtual DOM
- Advantages and Disadvantages of ReactJS
- Advantages of ReactJS
- Disadvantages of ReactJS
- Basic Knowledge You Need to Build Applications with React
- What is JSX?
- Components in React
- Props and State
- React Lifecycle
- Real-World Applications of ReactJS
- Single Page Applications (SPA)
- Complex User Interfaces
- Mobile Applications with React Native
- SEO Optimization with Next.js
- Content Management Systems (CMS)
- Real-Time Applications
- E-commerce
- Conclusion
- Why Choose ReactJS?
- When Should You Use ReactJS?
React is an open-source JavaScript library developed by Facebook, specifically designed for building user interfaces (UI), especially for web applications. Introduced in 2013, React has quickly become one of the most popular tools in the web development space thanks to its powerful data handling capabilities and performance-optimized rendering.
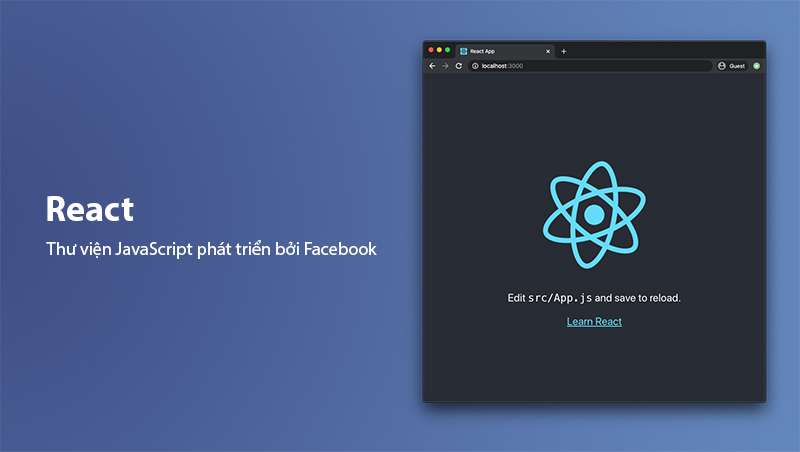
The standout feature of React is its Component-based architecture. With this approach, the UI is divided into smaller, manageable, and reusable components, helping developers save time and reduce errors during the application development process.
Another key aspect of React is the concept of the Virtual DOM (Document Object Model). This enables React to update the interface quickly and efficiently without reloading the entire webpage. This is why React is highly regarded for building Single Page Applications (SPA), where smooth user experience is a critical factor.
New to web development? Learn more about website optimization and useful tools to get started easily.
React is also an ideal choice for applications requiring good SEO, thanks to its ability to integrate with tools like Next.js to create Server-Side Rendering (SSR) or Static Site Generation (SSG) applications, improving search engine rankings. As a result, React is widely used not only in personal projects but also by large enterprises such as Netflix, Airbnb, and Uber.
If you’re looking for a flexible and powerful tool to develop modern web applications, React is a top choice. Let’s dive into the detailed features and applications of ReactJS in the following sections of this article.
Key Components in ReactJS
To better understand the power of React, you need to master the core components that make up its ecosystem. Here are the most important aspects:
Components
A Component is the building block of React. A component can be thought of as a "piece" of the UI, from small elements like buttons to larger sections like forms or entire webpages. There are two main types:
- Functional Components: These are JavaScript functions that return JSX. They are popular due to their simplicity and their ability to work with React Hooks.
- Class Components: Built as classes, they support more complex features but are becoming less commonly used.
Example: A simple button in React can be written as follows:
function Button() {
return <button>Click Me</button>;
}
JSX (JavaScript XML)
JSX is a syntax extension of JavaScript that allows you to write code similar to HTML in React. This unique feature makes building UIs more intuitive.
const element = <h1>Hello, React!</h1>;
Props (Properties)
Props are used to pass data from a parent component to a child component. This makes components flexible and reusable.
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
State
State is where a component's dynamic data is stored. Unlike props, state can be modified by the component that owns it, allowing the UI to respond in real-time.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You have clicked {count} times!</p>
<button onClick={() => setCount(count + 1)}>Increase</button>
</div>
);
}
Hooks
React Hooks (introduced in version 16.8) provide powerful tools like useState
and useEffect
to manage state and lifecycle within functional components.
Virtual DOM
React leverages the Virtual DOM to optimize performance. When changes occur, the Virtual DOM compares the old and new versions, then updates only the modified parts on the real DOM.
These components are the foundation for building robust, scalable, and maintainable applications. In the next section, we’ll analyze the advantages and disadvantages of ReactJS to better understand why it is so beloved by the developer community.
Advantages and Disadvantages of ReactJS
ReactJS has become a popular tool in the frontend development community thanks to its powerful and flexible features. However, like any technology, React has both advantages and disadvantages. Let’s analyze them in detail:
Advantages of ReactJS
-
High performance with Virtual DOM
With Virtual DOM, React only updates the elements that have changed, optimizing performance and improving application speed, especially for large web applications. -
Component reuse
The component-based architecture allows developers to reuse code, reducing development and maintenance time. -
Large community and rich ecosystem
React has a strong developer community, with plenty of documentation, libraries, and tools available. This makes it easy to find solutions to problems or extend functionality with libraries like Redux, React Router, or Material-UI. -
React Hooks
Hooks provide a modern, simple, and easier way to manage state and complex features in functional components. -
High compatibility
React can integrate with other frameworks like Next.js to create SEO-optimized applications or easily connect to backends via APIs. -
Cross-platform development support
With React Native, you can develop mobile applications using the same React logic, minimizing effort when building applications for multiple platforms.
Are you exploring top frontend frameworks? Don’t miss the article Top Frontend Frameworks of 2024.
Disadvantages of ReactJS
-
Steep learning curve
React has a relatively steep learning curve, especially for beginners. Understanding JSX, Virtual DOM, and code organization can be challenging at first. -
UI-focused only
React is just a library for building user interfaces and does not provide full features like routing or complex state management (additional libraries like Redux or Context API are needed). -
Frequent updates
React is constantly being updated and changed, requiring developers to regularly update their knowledge, which can sometimes disrupt projects. -
Client-side rendering
Although React can be combined with tools like Next.js to improve SEO for ReactJS, client-side rendering inherently has limitations for non-optimized applications.
Advantages | Disadvantages |
---|---|
High performance with Virtual DOM | Steep learning curve |
Component reuse | UI-focused only, requires additional libraries |
Large community and rich ecosystem | Frequent updates requiring constant learning |
React Hooks are simple and powerful | Limitations of client-side rendering for SEO |
High compatibility and cross-platform development support | No specific disadvantages, but requires experience |
ReactJS delivers high performance, flexibility, and excellent scalability, but it requires users to have experience and be ready to adapt to constant changes. In the next section, we’ll dive into the basic knowledge you need to get started with ReactJS.
Basic Knowledge You Need to Build Applications with React
Before starting to work with ReactJS, there are some fundamental concepts you need to understand to easily grasp and apply this tool in your projects:
What is JSX?
JSX (JavaScript XML) is the syntax used to write UI code in React. It combines JavaScript and HTML, making UI descriptions more intuitive. However, it’s important to note that JSX is merely a convenient syntax and will be compiled into JavaScript using Babel.
// JSX
const element = <h1>Hello, React!</h1>;
// Equivalent to JavaScript
const element = React.createElement('h1', null, 'Hello, React!');
Components in React
React is built on a component-based architecture. A component is an independent UI block that can be reused across different parts of the application.
- Functional Components: Easy to write and integrate with React Hooks for state management.
- Class Components: Previously widely used but are now less common due to the introduction of Hooks.
Props and State
- Props (Properties): Used to pass data from a parent component to a child component. Props are immutable (cannot be changed).
- State: Stores local data within a component and can be changed to reflect updates in the UI.
Example of state using useState
:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You have clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Increase</button>
</div>
);
}
React Lifecycle
Understanding the lifecycle of a component helps you better manage states and effects in React. When using class components, you need to work with methods like:
componentDidMount()
componentDidUpdate()
componentWillUnmount()
Meanwhile, with functional components, you can use React Hooks like useEffect
to handle lifecycle events.
React Hooks
Hooks like useState
, useEffect
, and useContext
have revolutionized the way React is written, eliminating the complexity of class components. This is one of the most crucial aspects to understand when working with modern React.
Once you grasp these basic concepts, you'll be ready to start building your first application. In the next section, we’ll explore real-world applications of ReactJS to better understand what React can offer.
Real-World Applications of ReactJS
ReactJS is widely used in various types of projects due to its flexibility and efficiency. Below are some common applications of ReactJS in real-world scenarios:
Single Page Applications (SPA)
React is a top choice for building SPAs, where pages don’t reload as users navigate between functionalities. This provides a fast, smooth user experience.
Examples: Gmail, Facebook, and Trello are notable SPAs built with React.
Complex User Interfaces
Thanks to its component-based architecture, React makes it easy to build complex user interfaces with high reusability. This is highly beneficial for large websites or management applications like CRMs or dashboards.
Mobile Applications with React Native
React isn’t just for the web! With React Native, you can use the same React logic to develop mobile applications for Android and iOS.
Examples: Mobile applications for Instagram, UberEats, and Airbnb are built with React Native.
SEO Optimization with Next.js
React integrates with Next.js to create Server-Side Rendering (SSR) or Static Site Generation (SSG) applications. This makes websites not only fast but also better optimized for search engines.
Examples: Many e-commerce websites like Shopify use React and Next.js to improve SEO and speed.
Content Management Systems (CMS)
React is often combined with Headless CMS (such as Contentful, Strapi) to create flexible, easily managed websites, particularly for large news sites or blogs.
Real-Time Applications
React is highly effective in building applications that require real-time updates, such as chat systems, notifications, or live data dashboards.
Examples: Slack and Discord leverage React to handle flexible UIs with large data volumes.
E-commerce
Many e-commerce platforms use React to create optimized user experiences with visually appealing interfaces and fast loading times.
Examples: Shopify, Amazon Prime Video.
React is not limited to simple websites but extends to mobile applications, e-commerce platforms, and large systems. In the next section, we’ll summarize the reasons why you should consider using ReactJS for your projects.
Conclusion
ReactJS has firmly established itself as one of the leading frontend libraries for building user interfaces. With powerful features like Virtual DOM, component-based architecture, and React Hooks, React simplifies the process of creating modern web applications, from Single Page Applications (SPA) to cross-platform mobile applications with React Native.
Why Choose ReactJS?
- Outstanding Performance: With its optimized DOM and fast UI handling, React ensures your application runs smoothly, even with large amounts of data.
- Flexibility: Easily integrates with other tools like Redux, Next.js, or any backend through APIs.
- Strong Community: Support from Meta (Facebook) and millions of developers worldwide ensures you always have learning resources and solutions when needed.
- Scalability: From small projects to complex systems, React handles it all well thanks to its modularity and reusability.
When Should You Use ReactJS?
React is suitable if you:
- Need to build a web or mobile application with a complex UI.
- Want to deliver fast, seamless user experiences.
- Require a library with a rich ecosystem and significant scalability.
If you’re considering starting with ReactJS, explore the official guides from ReactJS or experiment with small projects to understand how React works in practice. With strong community support and efficient optimization capabilities, ReactJS is undoubtedly an excellent choice for your development journey.
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
What is REST API? Complete A-Z Knowledge About REST API
REST API is one of the essential concepts that every backend developer needs to fully understand. This article provides comprehensive knowledge about REST API, including its definition, principles of operation, and how to build a standard RESTful API.
What is HATEOAS? How to Build APIs Using HATEOAS
Learn about HATEOAS, an important concept in API development, and how to build APIs using HATEOAS to improve interactivity and scalability.
What Is GraphQL? The Advantages of GraphQL Over REST API
Explore GraphQL, a modern API technology, and why it outperforms REST API in many web development scenarios.
What is XSS? Signs of Detection and Effective Prevention Methods
Learn about XSS, signs of detection, and effective prevention methods for XSS attacks in websites.
