Lesson 24. Converting Decimal to Binary in Java | Learn Basic Java
- Published on
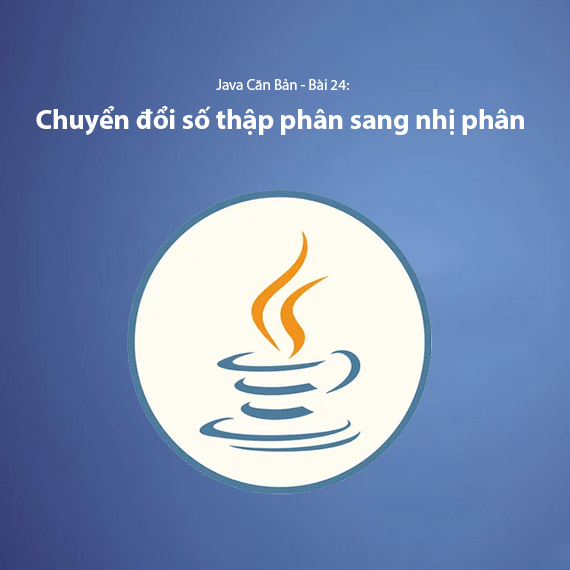
In programming, converting a number from the decimal system (base 10) to the binary system (base 2) is a common problem. Java provides several ways to perform this conversion, including:
- Using repeated division by 2 (manual algorithm).
- Using Java’s built-in method (
Integer.toBinaryString()
). - Using recursion.
This article will guide you through each method with detailed explanations and examples.
What are the Decimal and Binary Systems?
-
Decimal System (Base 10):
- The standard number system used in daily life.
- Consists of 10 digits: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9.
-
Binary System (Base 2):
- Uses only 2 digits: 0 and 1.
- Computers use the binary system to store and process data.
Example:
- 10 (decimal) → 1010 (binary).
- 25 (decimal) → 11001 (binary).
Method 1: Converting Decimal to Binary Using Repeated Division by 2
This method involves repeatedly dividing the decimal number by 2 and recording the remainder, then reading the remainders in reverse order.
Steps to Convert:
- Divide the decimal number by 2.
- Record the remainder.
- Continue dividing the quotient by 2 until it becomes 0.
- Read the remainders in reverse order to get the binary result.
Example: Convert 10 (decimal) to binary
Division | Quotient | Remainder |
---|---|---|
10 / 2 | 5 | 0 |
5 / 2 | 2 | 1 |
2 / 2 | 1 | 0 |
1 / 2 | 0 | 1 |
Result (read in reverse):
1010
(binary)
Java Implementation
import java.util.Scanner;
public class DecimalToBinary {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a decimal number: ");
int decimal = scanner.nextInt();
scanner.close();
System.out.println("Binary equivalent: " + convertToBinary(decimal));
}
public static String convertToBinary(int decimal) {
StringBuilder binary = new StringBuilder();
while (decimal > 0) {
int remainder = decimal % 2;
binary.insert(0, remainder); // Insert remainder at the beginning
decimal /= 2;
}
return binary.length() > 0 ? binary.toString() : "0"; // Return "0" if input is 0
}
}
Explanation:
- Uses a
while
loop to repeatedly divide the number by 2. - Uses
StringBuilder.insert(0, remainder)
to build the binary number from left to right. - Handles the case where input is 0 and returns
"0"
.
Integer.toBinaryString()
Method
Method 2: Using Java’s Built-in Java provides the built-in method Integer.toBinaryString(int number)
, making the conversion very simple.
Java Implementation
import java.util.Scanner;
public class DecimalToBinaryBuiltIn {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a decimal number: ");
int decimal = scanner.nextInt();
scanner.close();
// Using Java's built-in method
String binary = Integer.toBinaryString(decimal);
System.out.println("Binary equivalent: " + binary);
}
}
Explanation:
- Extremely simple and efficient.
- Uses Java’s built-in library, eliminating the need for a manual algorithm.
Method 3: Using Recursion to Convert Decimal to Binary
Recursion can also be used to convert a decimal number to binary.
Java Implementation
import java.util.Scanner;
public class DecimalToBinaryRecursive {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a decimal number: ");
int decimal = scanner.nextInt();
scanner.close();
System.out.println("Binary equivalent: " + convertToBinary(decimal));
}
public static String convertToBinary(int decimal) {
if (decimal == 0) {
return "0";
} else if (decimal == 1) {
return "1";
}
return convertToBinary(decimal / 2) + (decimal % 2);
}
}
Explanation:
- Uses recursion until the number is reduced to 0 or 1.
- Builds the binary number by concatenating the remainder to the result.
Comparison of Methods
Method | Time Complexity | Ease of Understanding | Performance |
---|---|---|---|
Repeated Division (Loop) | O(log N) | Easy | Fast |
Using | O(1) | Easiest | Fastest |
Using Recursion | O(log N) | More difficult | Slower for large numbers |
Conclusion
- Use the
while
loop method if you want to understand the algorithm. - Use
Integer.toBinaryString()
for the simplest and most efficient solution. - Use recursion if you want to practice recursion techniques.
In real-world applications, Integer.toBinaryString()
is the best choice due to its simplicity and performance!
Next article: Lesson 25:
do-while
Loop in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Lesson 22. How to Use For-Each Loop in Java | Learn Basic Java
Learn how to use the for-each loop in Java with syntax, practical examples, and its applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Lesson 22. How to Use For-Each Loop in Java | Learn Basic Java
Learn how to use the for-each loop in Java with syntax, practical examples, and its applications in Java programming.
