Lesson 22. Guide to Using For-Each Loop in Java | Learn Basic Java
- Published on
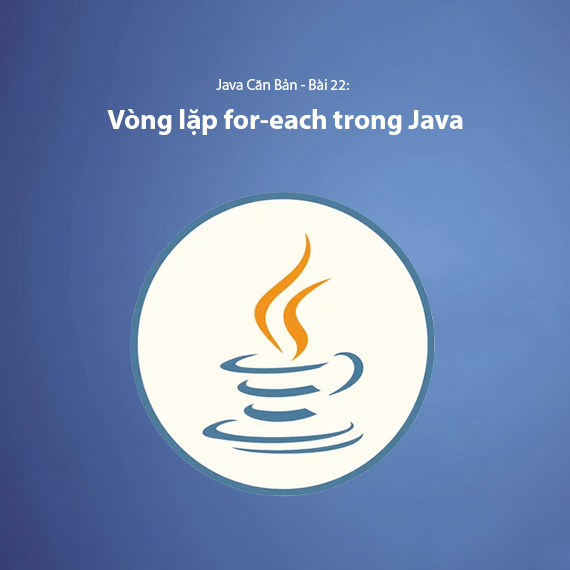
The for-each loop in Java is a way to iterate through elements in an array or a list without needing to worry about indices. It provides a concise syntax, making the code more readable and less error-prone compared to the traditional for
loop.
In this article, we will explore the syntax, functionality, advantages, disadvantages, and examples of the for-each loop in Java.
What is a For-Each Loop?
The for-each loop (also known as the enhanced for loop) is a special type of loop in Java used to iterate through each element in an array (array
) or a collection (Collection
).
Key differences compared to the traditional for loop:
- No need to know the size of the array or list.
- No need to use a counter variable (
i
). - Simple and easy-to-understand syntax.
Syntax of the For-Each Loop in Java
The syntax of the for-each loop is as follows:
for (DataType temporary_variable : collection) {
// Block of code to process each element
}
Explanation:
DataType
is the type of the elements in the collection.temporary_variable
is the variable used to store each element during iteration.collection
is the array or list you want to iterate through.
Examples of the For-Each Loop
Iterating Through an Integer Array
public class ForEachExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
System.out.println("Value: " + num);
}
}
}
Output:
Value: 1
Value: 2
Value: 3
Value: 4
Value: 5
Iterating Through a String Array
public class ForEachExample {
public static void main(String[] args) {
String[] names = {"Hà", "Nam", "Lan", "Tùng"};
for (String name : names) {
System.out.println("Name: " + name);
}
}
}
Output:
Name: Hà
Name: Nam
Name: Lan
Name: Tùng
Iterating Through an ArrayList
import java.util.ArrayList;
public class ForEachExample {
public static void main(String[] args) {
ArrayList<String> colors = new ArrayList<>();
colors.add("Red");
colors.add("Blue");
colors.add("Yellow");
for (String color : colors) {
System.out.println("Color: " + color);
}
}
}
Output:
Color: Red
Color: Blue
Color: Yellow
Comparison Between For-Each Loop and Traditional For Loop
Criteria | For-Each Loop | Traditional For Loop |
---|---|---|
Iteration Method | Automatically iterates through each element | Iterates using an index ( |
Code Length | Concise and easy to read | Longer and more complex |
Applicability | Arrays, lists | Arrays, lists, strings |
Ability to Modify Elements | ❌ No | ✅ Yes |
Access to Index | ❌ No | ✅ Yes |
When to use for-each?
- When you only need to iterate through each element without requiring the index.
- When you do not need to modify the values of elements in the list.
When to use traditional for loop?
- When you need to know the index of the element.
- When you need to modify the values of elements in the array or list.
Disadvantages of the For-Each Loop
Although the for-each loop has many advantages, it also has some limitations:
-
Cannot Modify Elements in the List
- When using the for-each loop, you cannot change the value of elements within the list.
❌ Example of Error:
int[] numbers = {1, 2, 3, 4, 5}; for (int num : numbers) { num = num * 2; // This change DOES NOT affect the original array } for (int num : numbers) { System.out.println(num); // Values remain unchanged }
-
Cannot Iterate in Reverse
- The for-each loop always iterates from the beginning to the end; it cannot iterate in reverse.
✅ If you need to iterate in reverse, use the traditional
for
loop:for (int i = numbers.length - 1; i >= 0; i--) { System.out.println(numbers[i]); }
-
Cannot Skip Elements or Conditionally Iterate
- The for-each loop iterates through all elements; it cannot skip elements based on a condition.
- If you need to skip elements, use the
for
orwhile
loop.
Summary
The for-each loop makes the code cleaner, but it is not always suitable. When you need to modify values or control the index, you should use the traditional for
loop.
Next Lesson: Lesson 23. How to Use the While Loop in Java Programming
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
