Lesson 23. Guide to Using the While Loop in Java | Learn Java Basics
- Published on
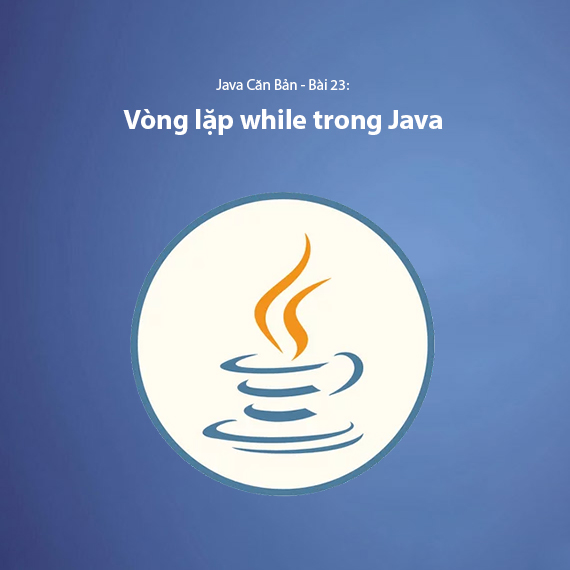
- What is the While Loop?
- When to Use the While Loop?
- Syntax of the While Loop in Java
- Explanation:
- How the While Loop Works
- Examples of the While Loop in Java
- Basic While Loop
- While Loop for Valid User Input
- Infinite While Loop (Exit on 'exit' Input)
- Advantages and Disadvantages of the While Loop
- Advantages:
- Disadvantages:
- Comparison Between While and Do-While Loops
- Conclusion
The while loop is one of the three main loop types in Java. It allows you to repeatedly execute a block of code as long as a condition remains true. This is an essential tool in programming, helping automate repetitive tasks without needing to write the same code multiple times.
What is the While Loop?
The while loop is a conditional loop in Java, meaning it continues looping as long as the condition remains true (true
). Once the condition becomes false
, the loop stops.
When to Use the While Loop?
- When you don’t know the exact number of iterations but only know the stopping condition.
- When you need to check the condition before executing the loop body.
- When you need an infinite loop with a stopping condition inside the loop.
Syntax of the While Loop in Java
while (condition) {
// Code block to be executed while the condition is true
}
Explanation:
condition
: A boolean expression that evaluates totrue
orfalse
.- If
condition
istrue
, the code inside{}
executes. - If
condition
isfalse
, the loop terminates immediately.
How the While Loop Works
- The condition (
condition
) is evaluated. - If the condition is
true
, the code inside{}
executes. - The condition is checked again.
- If the condition becomes
false
, the loop terminates.
Note: If the condition never becomes false, the loop runs indefinitely (infinite loop).
Examples of the While Loop in Java
Basic While Loop
Example: Printing numbers from 1 to 5.
public class WhileExample {
public static void main(String[] args) {
int i = 1; // Initialize counter
while (i <= 5) { // Check condition
System.out.println("Number: " + i);
i++; // Increment counter
}
}
}
Output:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
While Loop for Valid User Input
Example: Prompting the user to enter a number greater than 0.
import java.util.Scanner;
public class WhileExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int number;
System.out.print("Enter a number greater than 0: ");
number = scanner.nextInt();
while (number <= 0) {
System.out.print("Invalid number! Try again: ");
number = scanner.nextInt();
}
System.out.println("You entered: " + number);
scanner.close();
}
}
Output (if the user enters an incorrect value first):
Enter a number greater than 0: -3
Invalid number! Try again: 5
You entered: 5
Infinite While Loop (Exit on 'exit' Input)
Example: Continuously prompting the user for input until they type "exit"
:
import java.util.Scanner;
public class WhileExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String input;
while (true) { // Infinite loop
System.out.print("Enter a word (or 'exit' to quit): ");
input = scanner.nextLine();
if (input.equalsIgnoreCase("exit")) {
System.out.println("Exiting program.");
break; // Exit the loop
}
System.out.println("You entered: " + input);
}
scanner.close();
}
}
Output:
Enter a word (or 'exit' to quit): hello
You entered: hello
Enter a word (or 'exit' to quit): java
You entered: java
Enter a word (or 'exit' to quit): exit
Exiting program.
Advantages and Disadvantages of the While Loop
Advantages:
- Simple and easy to use when the number of iterations is unknown.
- Can run indefinitely if no stopping condition is provided.
- Efficient for handling user input validation.
Disadvantages:
- Can cause an infinite loop if the condition never changes.
- Less control over iterations compared to the
for
loop.
Comparison Between While and Do-While Loops
Feature | While Loop | Do-While Loop |
---|---|---|
Condition Checking | Before execution | After executing the loop body once |
Minimum Execution | 0 times (if the initial condition is | At least once |
When to Use? | When you need to check the condition first | When you need to execute the loop body at least once |
Conclusion
The while loop is a powerful tool in Java, allowing you to perform repetitive tasks when the number of iterations is unknown. Always ensure the loop condition updates properly to avoid infinite loops.
Next Lesson: Lesson 24. How to Convert Decimal to Binary in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 22. How to Use For-Each Loop in Java | Learn Basic Java
Learn how to use the for-each loop in Java with syntax, practical examples, and its applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 22. How to Use For-Each Loop in Java | Learn Basic Java
Learn how to use the for-each loop in Java with syntax, practical examples, and its applications in Java programming.
