Lesson 26. Using break, continue, and return in Java | Learn Java Basics
- Published on
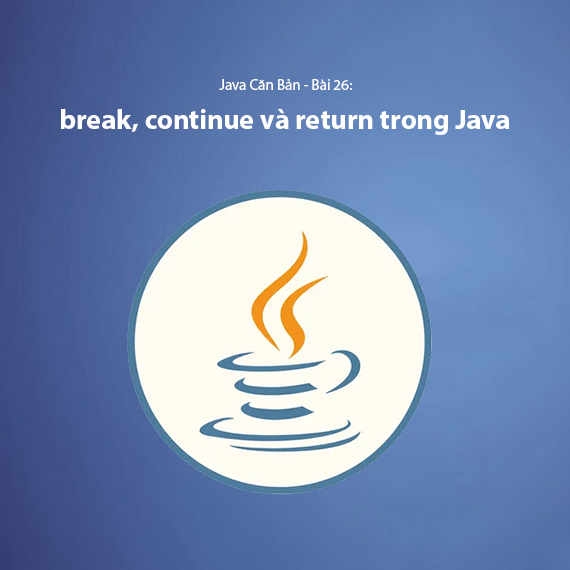
In Java programming, the break
, continue
, and return
statements are used to control the program's execution flow, especially within loops and methods.
break
is used to immediately exit a loop or aswitch-case
.continue
skips the current iteration and moves to the next loop iteration.return
immediately exits a method and can return a value.
This article provides a detailed guide on how to use these statements with practical examples.
break
Statement in Java
The break
in Loops
Using The break
statement stops a for
, while
, or do-while
loop immediately when a specified condition is met.
Example: Stop the loop when i == 5
:
public class BreakExample {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break; // Exit the loop when i = 5
}
System.out.println(i);
}
System.out.println("Loop has ended.");
}
}
Output:
1
2
3
4
Loop has ended.
When i == 5
, the break
statement is executed, terminating the for
loop immediately.
break
in switch-case
Using In switch-case
, break
prevents the program from executing subsequent cases after a match is found.
Example:
public class BreakSwitchExample {
public static void main(String[] args) {
int number = 2;
switch (number) {
case 1:
System.out.println("One");
break;
case 2:
System.out.println("Two");
break;
case 3:
System.out.println("Three");
break;
default:
System.out.println("Not recognized");
}
}
}
Output:
Two
Without break
, the program would continue executing the next cases.
break
with Labels
Using The break
statement can be used with a label to exit nested loops.
Example: Exit the entire loop when i == 2
and j == 2
:
public class BreakWithLabel {
public static void main(String[] args) {
outerLoop:
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
if (i == 2 && j == 2) {
break outerLoop; // Exit the outer loop
}
System.out.println("i = " + i + ", j = " + j);
}
}
System.out.println("Loop has ended.");
}
}
continue
Statement in Java
The continue
in Loops
Using The continue
statement skips the current iteration and moves to the next iteration of the loop.
Example: Skip when i == 5
:
public class ContinueExample {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
continue; // Skip this iteration
}
System.out.println(i);
}
}
}
Output:
1
2
3
4
6
7
8
9
10
When i == 5
, the program skips System.out.println(i);
and moves to i = 6
.
continue
with Labels
Using The continue
statement with a label skips the remaining loop body and jumps to the next iteration of the outer loop.
Example: When i == 2
and j == 2
, skip the rest of i
:
public class ContinueWithLabel {
public static void main(String[] args) {
outerLoop:
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
if (i == 2 && j == 2) {
continue outerLoop; // Skip the rest of the current iteration of i
}
System.out.println("i = " + i + ", j = " + j);
}
}
System.out.println("Loop has ended.");
}
}
return
Statement in Java
The return
in Methods
Using The return
statement is used to exit a method and optionally return a value.
Example: Calculate the sum of two numbers:
public class ReturnExample {
public static void main(String[] args) {
System.out.println("Sum of 3 and 4: " + calculateSum(3, 4));
}
public static int calculateSum(int a, int b) {
return a + b; // Return the sum
}
}
Output:
Sum of 3 and 4: 7
return
to Exit a Method Early
Using public class ReturnEarlyExample {
public static void main(String[] args) {
System.out.println(checkPositiveNumber(-5));
}
public static String checkPositiveNumber(int num) {
if (num <= 0) {
return "Invalid number"; // Exit immediately
}
return "Valid positive number";
}
}
return
in Loops
Using When return
is used inside a loop, the method exits immediately.
public class ReturnInLoop {
public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5};
System.out.println("Found number: " + findNumber(arr, 3));
}
public static int findNumber(int[] arr, int target) {
for (int num : arr) {
if (num == target) {
return num; // Return immediately when found
}
}
return -1; // Not found
}
}
In Java,
return
inside a loop will terminate the method that the loop is executing, rather than just exiting the loop likebreak
orcontinue
. Whenreturn
is called inside a loop, the program will exit the loop and immediately exit the method, returning a value (if applicable) to the caller of that method.
Conclusion
break
: Exits a loop when a condition is met.continue
: Skips the current iteration and moves to the next loop iteration.return
: Immediately exits a method.
Understanding these control flow statements helps improve your Java programming skills and optimize your code execution.
Latest Posts
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Lesson 22. How to Use For-Each Loop in Java | Learn Basic Java
Learn how to use the for-each loop in Java with syntax, practical examples, and its applications in Java programming.
Related Posts
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Lesson 22. How to Use For-Each Loop in Java | Learn Basic Java
Learn how to use the for-each loop in Java with syntax, practical examples, and its applications in Java programming.
