Lesson 25. The do-while Loop in Java | Learn Basic Java
- Published on
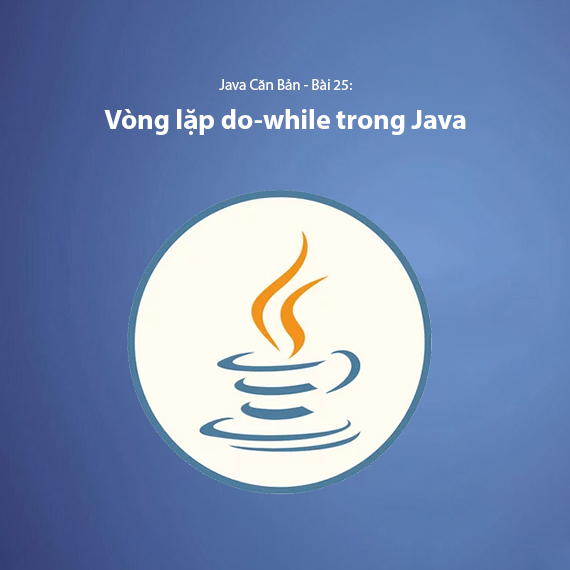
- What is the do-while loop?
- Syntax of the do-while loop in Java
- Basic Examples of the do-while Loop
- Example 1: do-while Loop with an Initially False Condition
- Example 2: do-while Loop for User Input Validation
- Comparison of do-while and while loops
- Key Differences:
- Real-World Applications of do-while Loop
- Prompting User Input Until Valid Data is Entered
- Calculating Sum Until the User Enters 0
- When Should You Use the do-while Loop?
- Conclusion
What is the do-while loop?
In Java programming, the do-while loop is one of the three fundamental loop types, alongside for
and while
. It is used when you want the block of code to execute at least once before checking the loop condition.
The do-while
loop has a simple syntax and is useful in many situations, such as prompting users for input until valid data is entered or performing an action at least once before evaluating whether to continue.
Syntax of the do-while loop in Java
The syntax of the do-while
loop in Java is as follows:
do {
// The block of code is executed at least once
} while (condition);
How the do-while loop works:
- The code inside
do
executes at least once. - After execution, the condition inside
while
is checked. - If the condition is true, the loop continues.
- If the condition is false, the loop terminates.
Basic Examples of the do-while Loop
Below are two examples of the do-while
loop:
- A
do-while
loop where the condition is false from the beginning - A
do-while
loop for validating user input
Example 1: do-while Loop with an Initially False Condition
Even though the condition is false (i < 5
is false at the start), the loop still executes at least once.
public class DoWhileWrongCondition {
public static void main(String[] args) {
int i = 10; // i is initialized with a value that does not meet the condition
do {
System.out.println("The do-while loop runs once!");
} while (i < 5); // Condition is false from the start
System.out.println("The do-while loop has ended.");
}
}
Output:
The do-while loop runs once!
The do-while loop has ended.
Explanation:
- Even though
i = 10
does not satisfyi < 5
, the loop still runs once before checking the condition. - After the first execution, the condition is false, so the loop terminates.
Example 2: do-while Loop for User Input Validation
The program prompts the user to enter a number between 1 and 10. If the input is incorrect, the user is asked to enter it again.
import java.util.Scanner;
public class DoWhileUserInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int number;
do {
System.out.print("Enter a number between 1 and 10: ");
number = scanner.nextInt();
if (number < 1 || number > 10) {
System.out.println("Invalid number! Please try again.");
}
} while (number < 1 || number > 10); // Condition check
System.out.println("You entered a valid number: " + number);
scanner.close();
}
}
Example program execution:
Enter a number between 1 and 10: 15
Invalid number! Please try again.
Enter a number between 1 and 10: 0
Invalid number! Please try again.
Enter a number between 1 and 10: 7
You entered a valid number: 7
Explanation:
- The program always runs at least once to prompt for input.
- If the number is outside the range of 1-10, the program asks for input again.
- When a valid number is entered, the loop stops and displays the result.
Comparison of do-while and while loops
Key Differences:
Feature | while | do-while |
---|---|---|
Condition checked first | Yes | No (executes first, checks later) |
Can run 0 times | Yes (if the condition is false initially) | No (always runs at least once) |
When to use? | When the loop might not need to execute | When at least one execution is required |
Real-World Applications of do-while Loop
Prompting User Input Until Valid Data is Entered
Suppose you want to ask the user for a number between 1 and 10, and if they enter an invalid number, they must try again.
import java.util.Scanner;
public class InputValidation {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int number;
do {
System.out.print("Enter a number between 1 and 10: ");
number = scanner.nextInt();
} while (number < 1 || number > 10); // Condition check
System.out.println("You entered a valid number: " + number);
scanner.close();
}
}
How it works:
- The loop runs at least once, prompting for input.
- If the entered number is out of range (1-10), the program asks for input again.
- When a valid number is entered, the loop stops and prints the valid input.
Calculating Sum Until the User Enters 0
import java.util.Scanner;
public class SumUntilZero {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int number;
int sum = 0;
do {
System.out.print("Enter a number (0 to stop): ");
number = scanner.nextInt();
sum += number; // Add input to total sum
} while (number != 0); // Stop when 0 is entered
System.out.println("Total sum of entered numbers: " + sum);
scanner.close();
}
}
How it works:
- The program asks for numbers multiple times.
- If the user enters
0
, the loop stops and prints the total sum.
When Should You Use the do-while Loop?
Use do-while
in situations like:
- When you need to ensure the block executes at least once.
- When prompting user input validation and requiring a valid entry.
- When handling menu-driven programs in console applications.
Avoid using do-while
when:
- You aren't sure if the loop should run at least once.
- You need to check the condition before executing the loop.
Conclusion
Feature | Details |
---|---|
Runs at least once? | Yes |
Comparison with |
|
When to use? | When at least one execution is needed before checking the condition |
Applications | Validating user input, console menus, condition checking |
In summary: The do-while
loop is useful when you need to execute the block at least once before checking the condition.
Next Article: Lesson 26: Using break, continue, and return in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Lesson 22. How to Use For-Each Loop in Java | Learn Basic Java
Learn how to use the for-each loop in Java with syntax, practical examples, and its applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Lesson 22. How to Use For-Each Loop in Java | Learn Basic Java
Learn how to use the for-each loop in Java with syntax, practical examples, and its applications in Java programming.
