What is REST API? Everything You Need to Know About REST API from A-Z
- Published on
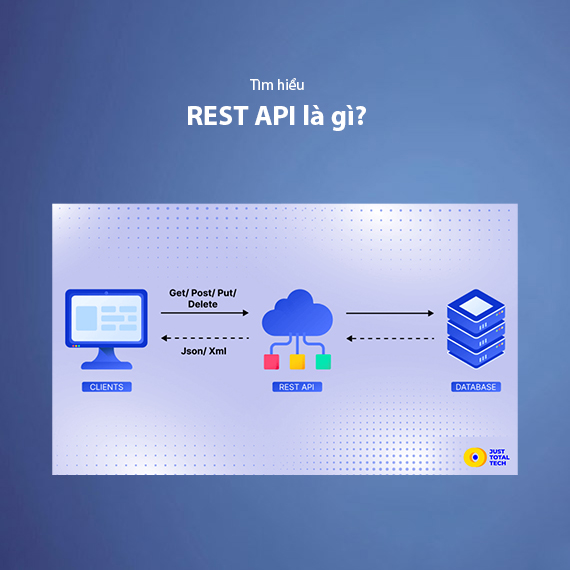
- What is REST API?
- The Importance of REST API
- Enabling Easy Communication Between Different Systems
- Supporting Multi-Platform Application Development
- Increasing System Flexibility and Scalability
- Basic Principles of REST API
- Client-Server Architecture
- Stateless
- Cacheable
- Uniform Interface
- Layered System
- Code on Demand (Optional)
- Key Components of REST API
- Resource
- HTTP Methods
- HTTP Status Codes
- Request and Response
- Advantages and Disadvantages of REST API
- Advantages of REST API
- Disadvantages of REST API
- Steps to Design a REST API
- Identify Resources and URI
- Choose the Appropriate HTTP Method
- Designing Response
- Error Handling
- Security
- Practical Examples of REST API
- Example of a Simple API
- Popular APIs
- Conclusion
What is REST API?
REST API (Representational State Transfer Application Programming Interface) is an application programming interface based on the REST (Representational State Transfer) architectural style, proposed by Roy Fielding in 2000. REST API allows applications to communicate with each other via the HTTP protocol, using standard methods like GET, POST, PUT, DELETE to query and manipulate data.
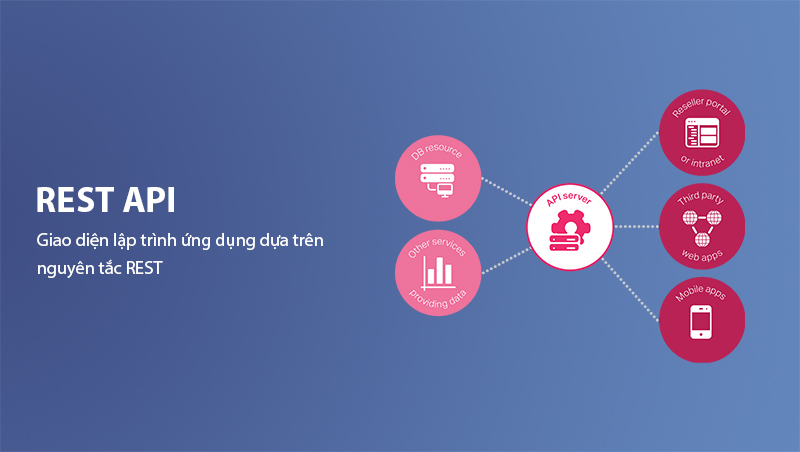
An important point about REST API is its consistency and simplicity. Instead of using complex protocols like SOAP, REST API takes full advantage of HTTP rules, making systems easier to scale and maintain. Today, REST APIs are widely used in web applications, mobile apps, microservices, and distributed systems.
For example, when you use an online shopping app, every time you search for a product, the app will send a request to the system's REST API to fetch a list of relevant products. The API will respond with data in JSON or XML format, helping the app display the results you need.
If you want to learn more about how to design APIs properly, you can refer to the article Guide to Building a RESTful API.
The Importance of REST API
Today, REST API plays a core role in software development, especially for web and mobile applications. With its flexibility, ease of use, and high performance, REST API has become the primary communication standard between different systems.
Enabling Easy Communication Between Different Systems
One of the biggest benefits of REST API is its ability to interact between different platforms. For example:
- A mobile app can send a request to the server via REST API to fetch product data and then display it to the user interface.
- A website can call an API from a third-party service, such as the Google Maps API, to integrate maps into the site.
- Internal systems within a company can use REST APIs to share data between departments without changing the existing system architecture.
Thanks to REST API, applications can communicate smoothly and efficiently, even if they are written in different programming technologies like Node.js, Python, Java, or PHP. This allows companies to easily scale their systems and integrate more services without facing technology barriers.
Supporting Multi-Platform Application Development
REST API helps developers build cross-platform applications easily and effectively. When using REST API, you can build web, mobile, and desktop applications without worrying about compatibility between systems. This is particularly important when developing multi-device applications.
For example, a company can develop a mobile app and a web app to provide services to customers. Instead of developing the entire backend system for each platform, they only need to build a single REST API, and both apps can communicate with this API to fetch data or perform operations.
Moreover, with the rise of microservices, REST APIs also help break down complex applications into smaller independent services, each focusing on a specific task. This increases flexibility in development and maintenance, as well as makes it easier to scale systems when needed.
You can learn more about microservices and how to design them in the article What is Microservices? A Basic Guide for Developers.
Increasing System Flexibility and Scalability
REST API provides the ability to scale and modify systems easily without interrupting existing services. Since REST APIs follow the stateless principle, each request from a client to a server is independent, allowing the system to scale as needed without being affected by previous states.
For example, a company might start with a simple REST API for a small application, but later expand the system to serve millions of users without facing performance or compatibility issues. Adding new features, integrating external services, or even upgrading backend software can all be done smoothly thanks to the flexible structure of REST API.
Additionally, applying load balancing and caching in REST API systems helps increase the ability to handle requests in high-load environments. REST API also allows you to build systems in the form of microservices, where each service can develop, deploy, and scale independently while easily integrating with other services.
Basic Principles of REST API
REST API operates based on a set of fundamental design principles to ensure consistency and efficiency. These principles not only make APIs easy to use but also enable systems to interact efficiently and scale easily. Below are the basic principles of REST API:
Client-Server Architecture
The client-server architecture is the first and most important principle of REST. In this model, the client and server are clearly separated. The client (user application) is only responsible for sending requests and displaying the results to the user, while the server (host) is responsible for processing data and returning the results.
This separation allows for independent development and maintenance. The client can change or evolve without affecting the server, and conversely, the server can change without affecting the client.
For example, a web application can communicate with a server through a REST API, while a mobile app can also connect to the same server through this API without changing the server.
Stateless
Another important principle of REST is stateless. This means that each request from the client to the server must contain all the information necessary for the server to process the request without relying on the state of previous requests.
This reduces storage overhead and makes it easier to scale the system. Every time the client sends a request, the server will process it without needing to rely on any information from previous requests.
For example, when you log into an app, each GET or POST request must include authentication details (like JWT token), as the server does not store previous login states.
Cacheable
The cacheable principle in REST API allows server responses to be stored (cached) on the client-side or between servers to improve performance and reduce load on the server. When a response is marked as cacheable, the client or intermediate devices can store this information and reuse it when similar requests occur, instead of having to send the request to the server every time.
This is particularly useful in applications that require data that doesn't change frequently, such as weather data, stock prices, or product listings. Caching helps reduce latency in loading content while decreasing server load.
For example, when you visit the homepage of an online store, featured products or promotions may be cached on the client or intermediate server, allowing them to load faster without needing to request them from the server every time.
Uniform Interface
One of the core principles of REST is the uniform interface. This means that the API must use a common protocol and approach to ensure that every request from the client to the server follows the same standard.
To maintain consistency in the API, HTTP methods like GET, POST, PUT, DELETE must be used uniformly, and resources (data entities) must be identified clearly via URLs. This helps developers understand and use the API easily, without needing to learn complex syntax or special rules.
For example, instead of having multiple endpoints like /create-user
or /update-product
, a standard RESTful API would use consistent endpoints like /users
for user-related actions (GET to fetch, POST to create, PUT to update) and /products
for product-related actions.
Layered System
One of the prominent features of REST API is the layered system principle. This principle allows a system to be divided into multiple layers, each responsible for a specific task, such as a layer for handling client requests, a layer for backend logic processing, or a layer for service provision.
With a layered architecture, systems can be easily expanded, maintained, and modified without affecting other parts of the system. This helps increase flexibility and reduce application complexity, as well as enhance security by placing protective layers between the client and server.
For example, in a complex REST API system, you might have a proxy or gateway layer responsible for authentication and access control before requests are forwarded to the actual backend services. This not only helps protect sensitive services but also enhances scalability and load handling.
Code on Demand (Optional)
Although it is an optional feature in REST, the code on demand principle can be applied in some special cases. When using this principle, the server can provide code to the client in certain situations. This code could be JavaScript scripts or configuration files that help the client perform certain tasks without needing additional server requests.
However, since this feature is optional, most modern RESTful APIs do not use it. When applied, code on demand can reduce load on the server and allow clients to perform dynamic tasks without constantly contacting the server.
For example, a web app might load a JavaScript snippet from the server to perform dynamic tasks like displaying maps or live search, without needing to send requests to the server each time the user types data.
Key Components of REST API
To understand how REST API works, we need to grasp the key components that REST API utilizes. These components not only help organize data and handle requests but also ensure that the API can scale and maintain consistency throughout its development.
Resource
In REST API, a resource is an object or a collection of data that can be accessed and manipulated via the API. A resource can be user data, products, blog posts, or any type of information managed by your system.
Each resource in a REST API is identified by a unique URL. For example, in a user management API, a user resource might be represented by a URL like https://api.example.com/users
. Here, users
is the resource that the client can interact with.
Operations such as GET (fetch), POST (create), PUT (update), and DELETE (delete) are performed on this resource.
HTTP Methods
To interact with resources, REST API uses standard HTTP methods as follows:
- GET: Used to fetch data from the server. For example,
GET /users
will return a list of all users. - POST: Used to create a new resource on the server. For example,
POST /users
will create a new user. - PUT: Used to update an existing resource. For example,
PUT /users/1
will update the user with ID 1. - DELETE: Used to delete a resource from the server. For example,
DELETE /users/1
will delete the user with ID 1.
Each of these methods has a specific purpose and usage, making the API powerful and easy to use.
HTTP Status Codes
HTTP status codes are an essential part of REST APIs. They indicate the result of a request made by the client to the server. Some common status codes include:
- 200 OK: The request was successful, and the server returned data (typically used for GET requests).
- 201 Created: The request was successful, and a new resource has been created (typically used for POST requests).
- 400 Bad Request: The request is invalid (usually due to client errors, such as missing parameters).
- 404 Not Found: The resource does not exist (the client requested a resource that is not available on the server).
- 500 Internal Server Error: An error occurred on the server while processing the request.
HTTP status codes help the client understand the result of the request and know how to handle issues when they arise.
Learn more about how to use HTTP Methods and Status Codes in the article What is HTTP Status Code and How to Use It.
Request and Response
In REST APIs, request and response are the two fundamental components that allow the client and server to communicate with each other. Every time a request is sent from the client, the server processes it and returns an appropriate response. These two components help define the information the client requests and how the results are returned from the server.
-
Request: An HTTP request from the client to the server includes key components like method (GET, POST, PUT, DELETE), URL (the address of the resource), and headers (additional information like data format, authentication tokens) along with body (the data being sent, if applicable). For example, when sending a POST request to create a new resource, data is included in the body.
- Example of a GET request:
GET /users/1 HTTP/1.1 Host: api.example.com
- Example of a POST request:
POST /users HTTP/1.1 Host: api.example.com Content-Type: application/json Body: { "name": "John Doe", "email": "john@example.com" }
- Example of a GET request:
-
Response: When the server receives the request, it processes it and sends back a response. The response includes components like status code (HTTP status code), headers (information about the type of data returned), and body (the content returned). The body content can include the requested data, error messages, or other information.
- Example of a response with status code 200 OK:
HTTP/1.1 200 OK Content-Type: application/json Body: { "id": 1, "name": "John Doe", "email": "john@example.com" }
- Example of a response with status code 404 Not Found:
HTTP/1.1 404 Not Found Body: { "error": "User not found" }
- Example of a response with status code 200 OK:
Through this process, REST APIs allow the client and server to exchange data in a flexible, easy-to-understand manner while ensuring that requests are handled and results are returned clearly and accurately.
Advantages and Disadvantages of REST API
When building and using a REST API, developers need to understand its advantages and disadvantages to make the most out of REST API in their projects.
Advantages of REST API
-
Simple and Easy to Use
REST APIs follow simple and understandable rules, making them easy for developers to learn and use. With standard HTTP methods (GET, POST, PUT, DELETE) and the use of URLs to identify resources, REST APIs can save development time and reduce errors in design. -
Good Scalability
Due to principles like stateless and layered systems, REST APIs are easily scalable as the system grows without encountering complex issues. By using REST APIs, you can easily add new features, services, or modify the backend structure without interrupting existing features. -
High Compatibility
One of the key advantages of REST APIs is their high compatibility. RESTful APIs can work across many different platforms, from mobile applications to large backend systems. This makes it easier to build cross-platform applications without worrying about compatibility issues between technologies. -
High Performance with Caching
REST APIs support caching to reduce the load on the server and increase processing speed. Responses can be cached at the client side or by intermediary servers, helping to reduce latency and improve user experience.
Disadvantages of REST API
-
Not Suitable for Complex Tasks
While REST API is great for simple CRUD (Create, Read, Update, Delete) tasks, it may not be the best solution when handling more complex tasks. For example, if you need to perform tasks that involve complex relationships between multiple resources, REST can introduce complexity and maintenance challenges. -
No Built-in State Management
REST is a stateless model, meaning the server does not store any information about the state of the client between requests. This can be challenging when managing sessions or tasks that require maintaining state throughout the user's experience. Solutions like JWT (JSON Web Token) can help address this issue, but they also add complexity to the design. -
Does Not Support Complex Operations (Limited Flexibility)
While REST is very flexible for basic operations, it lacks powerful features like query protocols to perform complex queries or multi-step operations. This makes RESTful APIs difficult to handle tasks that involve complex computations or large data sets.
Learn more about GraphQL and its pros and cons at https://riverlee/en/blog/what-is-graphql-la-gi.
Steps to Design a REST API
Designing an effective REST API requires following specific steps and standards to ensure the API is not only easy to use but also powerful and flexible. Here are the basic steps in the process of designing a REST API:
Identify Resources and URI
The first step in designing a REST API is to identify the resources you want your API to provide. Resources could be objects or data that the client can request or manipulate. These resources need to be identified by clear and understandable URIs (Uniform Resource Identifier).
For example, in a user management application, resources could be users, posts, or comments. The URI for these resources might look like this:
GET /users
: Retrieve a list of usersGET /users/{id}
: Retrieve user information by IDPOST /users
: Create a new userPUT /users/{id}
: Update user informationDELETE /users/{id}
: Delete a user
Ensuring that each resource is identified consistently will make using the API easier for developers.
Choose the Appropriate HTTP Method
After identifying the resources and URIs, the next step is to choose the appropriate HTTP method for each request. As previously mentioned, the basic HTTP methods in REST include GET, POST, PUT, and DELETE. Each method serves a different purpose:
- GET: Retrieve data from the server without changing the state of the resource.
- POST: Create a new resource on the server.
- PUT: Update an existing resource on the server.
- DELETE: Remove a resource from the server.
Choosing the right method helps keep the API understandable and adheres to RESTful standards, minimizing confusion when using the API.
Designing Response
A response is the data that the server returns to the client after processing the request. Designing the response in a logical way is crucial to ensure the usability and efficiency of the API. Each response should include the following components:
- Status code: The HTTP status code that indicates the result of the request (e.g., 200 OK, 404 Not Found, 500 Internal Server Error).
- Headers: Information about the returned data, such as content type (Content-Type).
- Body: The content of the returned data, which can be in formats such as JSON, XML, or others.
For example, when returning user information, the response could look like this:
HTTP/1.1 200 OK
Content-Type: application/json
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
}
Error Handling
When designing a REST API, error handling is an essential part. The API must return the appropriate error code and a clear message when an error occurs. This helps the client understand the cause of the issue and how to resolve it.
- 400 Bad Request: Invalid request from the client.
- 401 Unauthorized: The client does not have permission to access the resource.
- 403 Forbidden: The client is not allowed to access the resource, even after authentication.
- 404 Not Found: The resource does not exist.
- 500 Internal Server Error: An error occurred on the server while processing the request.
Example of a 404 Not Found error:
HTTP/1.1 404 Not Found
{
"error": "User not found"
}
Security
Security is a critical factor when designing a REST API. You need to ensure that your API not only functions effectively but also remains secure against external attacks. Some common security methods for APIs include:
- Authentication: Using methods like OAuth, JWT (JSON Web Tokens) to authenticate users and grant access rights.
- Encryption: Using SSL/TLS to encrypt data during transmission between the client and the server.
- Authorization: Determining different access rights for different types of users, ensuring that only authorized users can perform specific actions.
Practical Examples of REST API
To better understand how REST API works, we can look at some real-world examples of popular APIs today, as well as how REST API is used in actual applications.
Example of a Simple API
Suppose you are building a book management system in a library. The resources in the system could include books, authors, and book genres. Here are the endpoints and HTTP methods that could be used in your API:
GET /books
: Retrieve the list of all books in the library.GET /books/{id}
: Retrieve detailed information about a book based on its ID.POST /books
: Create a new book in the library.PUT /books/{id}
: Update the information of a book.DELETE /books/{id}
: Delete a book from the library.
An example of the GET /books request could return a list of books in JSON format:
HTTP/1.1 200 OK
Content-Type: application/json
[
{
"id": 1,
"title": "The Great Gatsby",
"author": "F. Scott Fitzgerald",
"genre": "Fiction"
},
{
"id": 2,
"title": "1984",
"author": "George Orwell",
"genre": "Dystopian"
}
]
Popular APIs
Many major services today use RESTful APIs to provide features to users and third-party applications. Here are a few popular APIs that you might have used or will need to work with in real projects:
- Twitter API: Provides access to tweets, followers, and other social networking features. Twitter uses REST API to return tweet information, post new tweets, follow others, etc.
- GitHub API: Allows users to access repositories, commits, issues, pull requests, and much more information from the GitHub platform.
- Google Maps API: Provides map services, directions, location searches, and other features that mobile or web applications can integrate.
Each of these APIs uses REST to allow users and other applications to easily and consistently access and manipulate data.
Conclusion
REST API is a powerful and flexible tool that helps systems communicate with each other in an easy and efficient manner. With basic principles like stateless, client-server architecture, and caching, REST API provides a simple and standardized approach to building web services.
When designing a REST API, correctly identifying resources, selecting the appropriate HTTP methods, and clearly designing responses are crucial to ensuring that your API is easy to use, maintain, and scale. At the same time, security is an essential factor to ensure the safety of data and applications.
With real-world examples such as the Twitter and GitHub APIs, you can clearly see the scalability and flexibility of REST API in large applications. Hopefully, this article has helped you understand more about how to build and use REST APIs in your software projects.
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
What is HATEOAS? How to Build APIs Using HATEOAS
Learn about HATEOAS, an important concept in API development, and how to build APIs using HATEOAS to improve interactivity and scalability.
What Is GraphQL? The Advantages of GraphQL Over REST API
Explore GraphQL, a modern API technology, and why it outperforms REST API in many web development scenarios.
What is XSS? Signs of Detection and Effective Prevention Methods
Learn about XSS, signs of detection, and effective prevention methods for XSS attacks in websites.
What is CSS? Why is CSS Essential in Web Design?
Learn about the concept of CSS and why it is an indispensable element in designing and optimizing website interfaces.
