Lesson 10. A Guide to Data Type Conversion in Java | Learn Basic Java Programming
- Published on
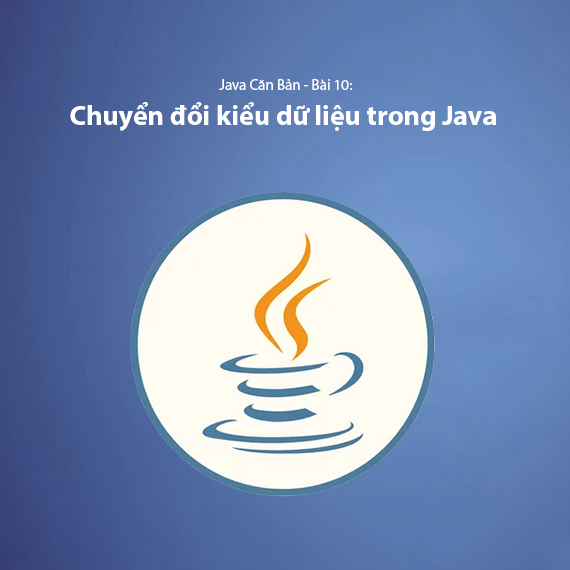
- What is Data Type Conversion in Java?
- Implicit Casting
- Explicit Casting
- Conversion between Primitive Data Types and Object Types
- Converting from Primitive Type to Object (Boxing)
- Converting from Object to Primitive Type (Unboxing)
- Autoboxing and Auto-unboxing
- Conversion between Numeric Types and String Types
- Converting a Number to a String
- Converting a String to a Number
- Conversion between Reference Data Types (Classes and Objects)
- Converting from Child Class to Parent Class (Upcasting)
- Downcasting (Converting from Parent Class to Child Class)
- Conclusion:
- Practical Exercise
What is Data Type Conversion in Java?
Data Type Conversion (Type Casting) in Java is the process of changing the type of a value from one data type to another. Java supports various types of type conversions, including:
- Implicit Casting
- Explicit Casting
- Conversion between primitive data types and object types (Boxing and Unboxing)
- Conversion between numeric types and strings (String)
- Conversion between reference data types (Reference Type Casting)
Implicit Casting
Implicit Casting (also known as Widening Casting) occurs when Java automatically converts a value from a smaller data type to a larger data type without data loss.
Smaller Type | Can be converted to |
---|---|
byte | short , int , long , float , double |
short | int , long , float , double |
int | long , float , double |
long | float , double |
float | double |
Example:
public class ImplicitCastingExample {
public static void main(String[] args) {
int myInt = 100;
double myDouble = myInt; // Automatically converts from int to double
System.out.println("Value of int: " + myInt);
System.out.println("Value of double: " + myDouble);
}
}
- The variable
myInt
has the typeint
and is assigned the value 100. - The variable
myDouble
has the typedouble
, and it receives the value frommyInt
without needing explicit casting. - Java automatically converts from
int
todouble
without data loss.
Explicit Casting
Explicit Casting (also known as Narrowing Casting) usually occurs when we convert from a larger data type to a smaller one. This requires the programmer to explicitly specify the desired type using parentheses.
In practice, explicit casting does not depend on whether the destination type is larger or smaller. As long as you use parentheses (type) to request the type conversion, it is always considered explicit casting, regardless of whether the destination type is larger or smaller than the original type.
Larger Type | Can be converted to |
---|---|
double | float , long , int , short , byte |
float | long , int , short , byte |
long | int , short , byte |
int | short , byte |
short | byte |
Example:
public class ExplicitCastingExample {
public static void main(String[] args) {
double myDouble = 9.78;
int myInt = (int) myDouble; // Convert from double to int
System.out.println("Original double value: " + myDouble);
System.out.println("Value after casting to int: " + myInt);
}
}
- The variable
myDouble
has the typedouble
with a value of 9.78. - When converting
double
toint
, the decimal part is lost (9.78 becomes 9). - You need to explicitly specify the type
(int) myDouble
to tell Java that you want to convert fromdouble
toint
.
Conversion between Primitive Data Types and Object Types
Java provides Wrapper Classes like Integer, Double, Float, etc., to convert between primitive data types and objects.
Converting from Primitive Type to Object (Boxing)
public class BoxingExample {
public static void main(String[] args) {
int num = 100;
Integer obj = Integer.valueOf(num); // Boxing (converting from int to Integer)
System.out.println("Integer object value: " + obj); // Integer object value: 100
}
}
- The
Integer.valueOf(num)
method creates an Integer object from the int value. - At this point,
obj
is anInteger
object containing the value 100. When printed, thetoString()
method of the Integer class is automatically called, and its value will be displayed as 100.
Converting from Object to Primitive Type (Unboxing)
public class UnboxingExample {
public static void main(String[] args) {
Integer obj = 50; // Autoboxing (automatic boxing)
int num = obj.intValue(); // Unboxing (converting from Integer to int)
System.out.println("int value: " + num);
}
}
obj.intValue()
performs the unboxing process, which converts theInteger
object to its primitiveint
value.- Here,
obj
is anInteger
object, and theintValue()
method helps retrieve the primitive value 50 from this object.
Autoboxing and Auto-unboxing
Java provides an automatic mechanism to perform boxing and unboxing, called autoboxing and auto-unboxing. This allows us not to explicitly call methods like valueOf()
or intValue()
in simple cases.
Example:
public class AutoBoxingExample {
public static void main(String[] args) {
int num = 100;
Integer obj = num; // Autoboxing (automatically converts from int to Integer)
System.out.println("Integer object value: " + obj);
int num2 = obj; // Auto-unboxing (automatically converts from Integer to int)
System.out.println("int value: " + num2);
}
}
Conversion between Numeric Types and String Types
Converting a Number to a String
You can use the String.valueOf()
method or the +""
operator to convert a number to a string.
Example:
public class NumberToString {
public static void main(String[] args) {
int num = 123;
String str1 = String.valueOf(num);
String str2 = num + ""; // Or use the + operator
System.out.println("String from int: " + str1);
System.out.println("String from int (method 2): " + str2);
}
}
Converting a String to a Number
Use methods like Integer.parseInt()
, Double.parseDouble()
, Float.parseFloat()
, etc.
Example:
public class StringToNumber {
public static void main(String[] args) {
String str = "456";
int num = Integer.parseInt(str);
double dnum = Double.parseDouble(str);
System.out.println("Integer from string: " + num);
System.out.println("Double from string: " + dnum);
}
}
Conversion between Reference Data Types (Classes and Objects)
Java supports type conversion between parent and child classes when using inheritance extends
.
- Upcasting: Converting from a child class to a parent class (automatically).
- Downcasting: Converting from a parent class to a child class (requires explicit casting).
Converting from Child Class to Parent Class (Upcasting)
- Automatically performed if the child class extends the parent class.
- No explicit casting required.
Example:
class Animal {
void makeSound() {
System.out.println("Some sound...");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Woof woof!");
}
}
public class UpcastingExample {
public static void main(String[] args) {
Animal myDog = new Dog(); // Upcasting from Dog → Animal
myDog.makeSound();
}
}
Downcasting (Converting from Parent Class to Child Class)
- Explicit casting is required.
- Can cause a
ClassCastException
if not checked beforehand.
Example:
public class DowncastingExample {
public static void main(String[] args) {
Animal myAnimal = new Dog(); // Upcasting
Dog myDog = (Dog) myAnimal; // Downcasting (explicit casting)
myDog.bark();
}
}
To be sure, you should check before casting using instanceof
to avoid errors.
if (myAnimal instanceof Dog) {
Dog myDog = (Dog) myAnimal;
myDog.bark();
}
Conclusion:
Type casting in Java is an important concept that helps developers work flexibly with different types of data. Java supports two main methods of type conversion: Implicit Casting and Explicit Casting.
- Implicit Casting (Widening Casting) happens when Java automatically converts a value from a smaller data type to a larger one without any intervention from the programmer.
- Explicit Casting (Narrowing Casting) requires the programmer to explicitly specify the target type, as this conversion may lose data (e.g., converting from
double
toint
).
Additionally, Java provides Wrapper Classes like Integer
, Double
, Float
, etc., to support the conversion between primitive types and object types through Boxing (converting from primitive type to object) and Unboxing (converting from object to primitive type).
Type casting also occurs in techniques like Upcasting and Downcasting in inheritance, allowing flexibility when using child class objects through parent class references while still being able to execute methods specific to the actual object type.
Type of Conversion | Description | Example |
---|---|---|
Implicit Casting (Widening Casting) | Converts from a smaller data type to a larger data type without requiring programmer intervention. | int → long, float → double. |
Explicit Casting (Narrowing Casting) | Converts from a larger data type to a smaller one. It must be explicitly stated and may lose data. | double → int, long → short. |
Boxing (Converting from Primitive Type to Object) | Converts from a primitive type to an object (Wrapper Class). | int → Integer, double → Double. |
Unboxing (Converting from Object to Primitive Type) | Converts an object (Wrapper Class) back to the corresponding primitive type. | Integer → int, Double → double. |
Upcasting (Converting from Child Class to Parent Class) | The process of converting a child class object to a parent class reference, allowing the use of parent class methods. | Dog → Animal, Car → Vehicle. |
Downcasting (Converting from Parent Class to Child Class) | Converts an object from a parent class to a child class, which may cause errors if the type check is not performed first. | Animal → Dog, Vehicle → Car (must check type before downcast). |
Understanding and correctly applying type conversion techniques will help developers optimize their code and avoid data type errors during Java application development.
Practical Exercise
Write a program that takes a floating-point number double
as input, and then:
- Convert this floating-point number to
int
and print it. - Convert it to a string and display it.
- Convert the string back to an integer
int
and print it.
Next lesson: Lesson 11. Basic Operations in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
