Lesson 11. Basic Operators in Java | Learn Basic Java
- Published on
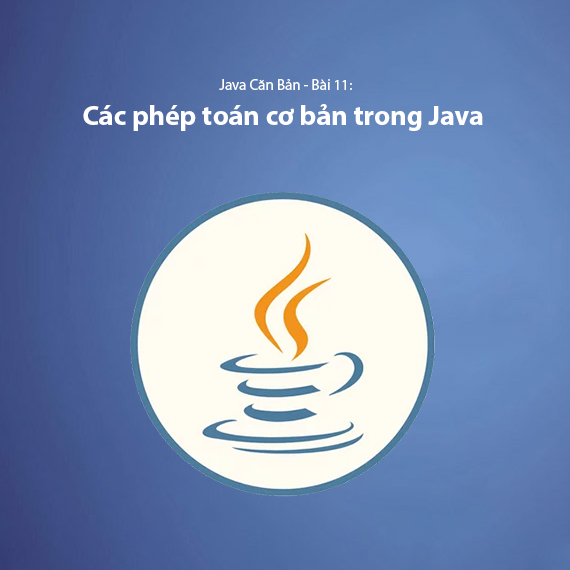
In Java, basic operators are fundamental operations that you will use to perform calculations in your programs. Java provides many types of operators to work with numerical values, strings, and other objects. In this article, we will explore the different types of basic operators in Java, including:
- Arithmetic operators
- Relational operators
- Logical operators
- Assignment operators
- Increment and Decrement operators
- Bitwise operators
Arithmetic Operators
Arithmetic operators are used to perform basic calculations such as addition, subtraction, multiplication, division, and remainder. In Java, arithmetic operations use the following symbols:
Operator | Symbol | Description |
---|---|---|
Addition | + | Adds two numbers together |
Subtraction | - | Subtracts two numbers |
Multiplication | * | Multiplies two numbers |
Division | / | Divides two numbers |
Remainder | % | Returns the remainder of division |
Example:
public class ArithmeticOperators {
public static void main(String[] args) {
int a = 10;
int b = 5;
System.out.println("Addition: " + (a + b)); // 10 + 5 = 15
System.out.println("Subtraction: " + (a - b)); // 10 - 5 = 5
System.out.println("Multiplication: " + (a * b)); // 10 * 5 = 50
System.out.println("Division: " + (a / b)); // 10 / 5 = 2
System.out.println("Remainder: " + (a % b)); // 10 % 5 = 0
}
}
The result when running the above program will be:
Addition: 15
Subtraction: 5
Multiplication: 50
Division: 2
Remainder: 0
Relational Operators
Relational operators are used to compare values and return true or false. The relational operators in Java include:
Operator | Symbol | Description |
---|---|---|
Equal to | == | Checks if two values are equal |
Not equal to | != | Checks if two values are not equal |
Greater than | > | Checks if the left value is greater than the right value |
Less than | < | Checks if the left value is less than the right value |
Greater than or equal to | >= | Checks if the left value is greater than or equal to the right value |
Less than or equal to | <= | Checks if the left value is less than or equal to the right value |
Example:
public class RelationalOperators {
public static void main(String[] args) {
int a = 10;
int b = 5;
System.out.println("a == b: " + (a == b)); // false
System.out.println("a != b: " + (a != b)); // true
System.out.println("a > b: " + (a > b)); // true
System.out.println("a < b: " + (a < b)); // false
System.out.println("a >= b: " + (a >= b)); // true
System.out.println("a <= b: " + (a <= b)); // false
}
}
The result when running the above program will be:
a == b: false
a != b: true
a > b: true
a < b: false
a >= b: true
a <= b: false
Logical Operators
Logical operators are used to perform operations with boolean values (true or false). Java provides three basic logical operators:
Operator | Symbol | Description |
---|---|---|
AND | && | Results in true if both expressions are true |
OR | || | Results in true if at least one expression is true |
NOT | ! | Negates the boolean value (true becomes false, and vice versa) |
Example:
public class LogicalOperators {
public static void main(String[] args) {
boolean a = true;
boolean b = false;
System.out.println("a && b: " + (a && b)); // false
System.out.println("a || b: " + (a || b)); // true
System.out.println("!a: " + !a); // false
}
}
The result when running the above program will be:
a && b: false
a || b: true
!a: false
Assignment Operators
Assignment operators are used to assign values to a variable. Java supports several special assignment operators, including:
Operator | Symbol | Description |
---|---|---|
Assign | = | Assign a value to a variable |
Add assignment | += | Assign value plus current value |
Subtract assignment | -= | Assign value minus current value |
Multiply assignment | *= | Assign value multiplied by current value |
Divide assignment | /= | Assign value divided by current value |
Remainder assignment | %= | Assign remainder of value with current value |
Example
public class AssignmentOperators {
public static void main(String[] args) {
int a = 10;
a += 5; // a = a + 5
System.out.println("a += 5: " + a); // 15
a -= 3; // a = a - 3
System.out.println("a -= 3: " + a); // 12
a *= 2; // a = a * 2
System.out.println("a *= 2: " + a); // 24
a /= 4; // a = a / 4
System.out.println("a /= 4: " + a); // 6
}
}
The result when running the above program will be:
a += 5: 15
a -= 3: 12
a *= 2: 24
a /= 4: 6
Increment and Decrement Operators
Increment and decrement operators are used to change the value of a variable by 1. Java provides two increment and decrement operators:
Operator | Symbol | Description |
---|---|---|
Pre-increment | ++a | Increments the value of a by 1 before using it |
Post-increment | a++ | Increments the value of a by 1 after using it |
Pre-decrement | --a | Decrements the value of a by 1 before using it |
Post-decrement | a-- | Decrements the value of a by 1 after using it |
Example:
public class IncrementDecrementOperators {
public static void main(String[] args) {
int a = 5;
System.out.println("++a: " + (++a)); // Increment first, a = 6
System.out.println("a++: " + (a++)); // Increment after, a = 6, but value is 6
System.out.println("a: " + a); // a = 7
System.out.println("--a: " + (--a)); // Decrement first, a = 6
System.out.println("a--: " + (a--)); // Decrement after, a = 6, but value is 6
System.out.println("a: " + a); // a = 5
}
}
The result when running the above program will be:
++a: 6
a++: 6
a: 7
--a: 6
a--: 6
a: 5
Bitwise Operators
Bitwise operators are used to perform bit-level operations on integer values. Bitwise operators include:
Operator | Symbol | Description |
---|---|---|
Bitwise AND | & | Bitwise AND operation |
Bitwise OR | | | Bitwise OR operation |
Bitwise XOR | ^ | Bitwise XOR operation |
Bitwise NOT | ~ | Inverts all bits |
Shift left | << | Shifts bits to the left |
Shift right | >> | Shifts bits to the right |
Example:
public class BitwiseOperators {
public static void main(String[] args) {
int a = 5; // 0101
int b = 3; // 0011
System.out.println("a & b: " + (a & b)); // 0001 -> 1
System.out.println("a | b: " + (a | b)); // 0111 -> 7
System.out.println("a ^ b: " + (a ^ b)); // 0110 -> 6
System.out.println("~a: " + (~a)); // 1010 -> -6
System.out.println("a << 1: " + (a << 1)); // 1010 -> 10
System.out.println("a >> 1: " + (a >> 1)); // 0010 -> 2
}
}
The result when running the above program will be:
a & b: 1
a | b: 7
a ^ b: 6
~a: -6
a << 1: 10
a >> 1: 2
Conclusion
The basic operators in Java are important tools that help you perform calculations in your program. You can use arithmetic operators, relational operators, logical operators, and many other operators to manipulate values and perform tasks in your applications. Understanding these operators well will help you write efficient and accurate code.
Next article: Article 12. Unary Operators in Java Programming
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
