Lesson 12. Unary Operators in Java | Learning Java Basics
- Published on
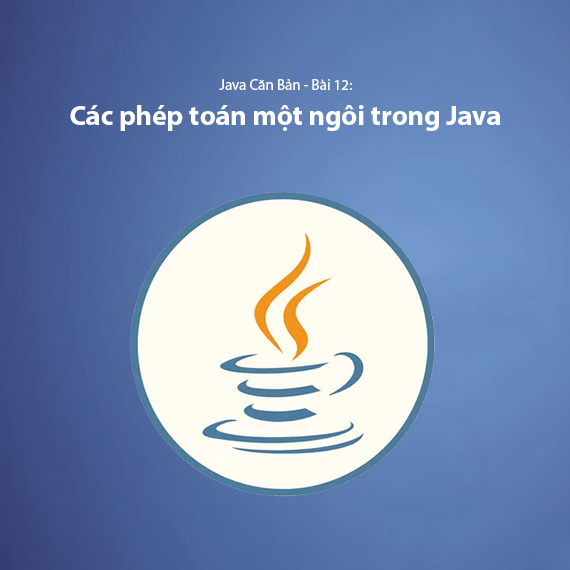
- What Are Unary Operators in Java?
- Unary Increment and Decrement Operators
- Increment Operator (Prefix and Postfix)
- Decrement Operator (Prefix and Postfix)
- Boolean Negation Operator (NOT)
- Other Unary Operators
- Negation Operator (-)
- Bitwise NOT Operator (~)
- Type Casting Operator ((type))
- Unary Operators and Practical Applications
- Using Unary Operators in Loops
- Using Unary Operators for Variable Updates
- Conclusion
What Are Unary Operators in Java?
In Java programming, Unary Operators are used to perform operations on a single operand. These operators are common and easy to use when modifying the value of a variable or performing simple operations.
Unary operators include:
- Increment operator (
++
) - Decrement operator (
--
) - Boolean negation operator (
!
) - Other unary operators such as the negation operator (
-
)
Let's explore them in detail below.
Unary Increment and Decrement Operators
Unary increment and decrement operators are used to modify the value of a primitive variable by 1. These operators are commonly used in loops or to update variables in programs.
Increment Operator (Prefix and Postfix)
-
Prefix increment:
++a
This operator increases the value of the variablea
by 1 before using its value in an expression. -
Postfix increment:
a++
This operator increases the value of the variablea
by 1 after using its value in an expression.
Example:
public class UnaryOperators {
public static void main(String[] args) {
int a = 5;
// Prefix increment
System.out.println("++a: " + (++a)); // Increments first, a = 6
// Postfix increment
System.out.println("a++: " + (a++)); // Uses value first, a = 6, then increments
System.out.println("a: " + a); // a = 7
}
}
Output:
++a: 6
a++: 6
a: 7
Decrement Operator (Prefix and Postfix)
-
Prefix decrement:
--a
This operator decreases the value of the variablea
by 1 before using its value in an expression. -
Postfix decrement:
a--
This operator decreases the value of the variablea
by 1 after using its value in an expression.
Example:
public class UnaryOperators {
public static void main(String[] args) {
int a = 5;
// Prefix decrement
System.out.println("--a: " + (--a)); // Decrements first, a = 4
// Postfix decrement
System.out.println("a--: " + (a--)); // Uses value first, a = 4, then decrements
System.out.println("a: " + a); // a = 3
}
}
Output:
--a: 4
a--: 4
a: 3
Boolean Negation Operator (NOT)
The negation operator (NOT) is used to invert the boolean value of a variable. This operator can change true
to false
and vice versa. In Java, the NOT operator is represented by !
.
- Boolean negation:
!a
Ifa
istrue
, then!a
returnsfalse
. Ifa
isfalse
, then!a
returnstrue
.
Example:
public class UnaryOperators {
public static void main(String[] args) {
boolean a = true;
boolean b = false;
System.out.println("!a: " + !a); // false
System.out.println("!b: " + !b); // true
}
}
Output:
!a: false
!b: true
Other Unary Operators
-
)
Negation Operator (In addition to the increment (++
) and decrement (--
) operators, Java also provides the negation operator (-
), which is used to change the sign of a number. This operator is particularly useful when you need to quickly switch between positive and negative values.
When applying -
before a number or numeric variable, its value is reversed:
- If the number is positive, it becomes negative.
- If the number is negative, it becomes positive.
Example:
public class UnaryOperatorExample {
public static void main(String[] args) {
int a = 5;
int b = -10;
System.out.println("Initial value of a: " + a);
System.out.println("Value of a after negation: " + (-a));
System.out.println("Initial value of b: " + b);
System.out.println("Value of b after negation: " + (-b));
}
}
Output:
Initial value of a: 5
Value of a after negation: -5
Initial value of b: -10
Value of b after negation: 10
~
)
Bitwise NOT Operator (The bitwise NOT operator (~
) is a unary operator in Java that works directly on the binary bits of an integer. This operator flips all bits in the binary representation of the number, meaning:
- Bit 1 becomes 0
- Bit 0 becomes 1
~x = -(x + 1)
This means that applying ~
to a number x
results in the inverted value of x, minus one.
Example:
public class BitwiseNotExample {
public static void main(String[] args) {
int num = 5; // 0000 0101 (5 in binary)
int result = ~num; // 1111 1010 (-6 in binary)
System.out.println("Initial value: " + num);
System.out.println("Result after using ~: " + result);
}
}
Output:
Initial value: 5
Result after using ~: -6
(type)
)
Type Casting Operator (The type casting operator ((type)
) is used to convert the data type of a variable from one type to another.
(type) variable
Where:
type
is the target data type.variable
is the variable to be converted.
Example:
public class TypeCastingExample {
public static void main(String[] args) {
double pi = 3.14;
int intPi = (int) pi; // intPi = 3
System.out.println("Initial value: " + pi);
System.out.println("Value after type casting: " + intPi);
}
}
Output:
Initial value: 3.14
Value after type casting: 3
Unary Operators and Practical Applications
Unary operators are widely used in programming, especially when handling loops or updating variable values. These operators help simplify code and efficiently perform simple operations.
Using Unary Operators in Loops
Increment and decrement operators are particularly useful for controlling loops. They allow us to modify the loop control variable efficiently.
Example:
public class UnaryOperators {
public static void main(String[] args) {
for (int i = 0; i < 5; ++i) {
System.out.println("i: " + i); // Increments i in each iteration
}
}
}
Using Unary Operators for Variable Updates
Increment and decrement operators help simplify code when updating a variable’s value without writing the full expression.
Example:
public class UnaryOperators {
public static void main(String[] args) {
int x = 10;
// Updating variable using unary operators
x++; // Increases x by 1
System.out.println("Value of x after increment: " + x); // 11
x--; // Decreases x by 1
System.out.println("Value of x after decrement: " + x); // 10
}
}
Output:
Value of x after increment: 11
Value of x after decrement: 10
Conclusion
Unary operators in Java, including increment, decrement, and negation operators, are powerful tools that allow programmers to modify variable values easily. They help simplify code and are useful in various scenarios, such as loops, variable updates, and boolean value handling. Understanding these operators will help you optimize your code and improve your Java programming efficiency.
We hope this article has helped you understand unary operators in Java. Try applying them in your exercises and projects to get familiar with their behavior!
Next article: Lesson 13. How to Assign Data in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
