Lesson 21. Guide to Using the For Loop in Java | Learn Basic Java
- Published on
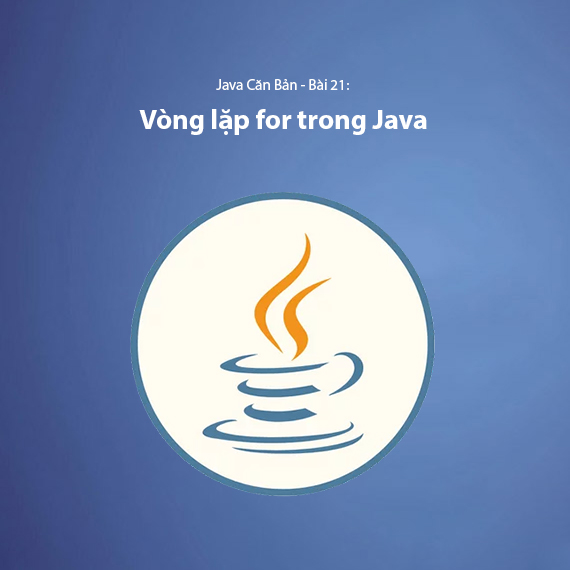
- What is the for Loop?
- Syntax of the for Loop
- Simple Example:
- Variations of the for Loop
- Using Multiple Control Variables
- Nested Loops
- Explanation:
- Omitting One or More Components
- Iterating Through an Array Using the for Loop
- The for-each Loop (Enhanced For Loop)
- Syntax of the for-each Loop
- Example:
- Using for with break and continue
- Using break to Exit the Loop Early
- Using continue to Skip an Iteration
- Conclusion
for
Loop?
What is the In programming, loops are an essential technique that allows you to execute a block of code repeatedly without having to write it multiple times. Java provides several types of loops, and the for
loop is one of the most commonly used.
The for
loop is used when you know in advance how many times the loop should run, making your code cleaner, more readable, and easier to maintain.
for
Loop
Syntax of the The general syntax of the for
loop in Java is as follows:
for (initialization; condition; update) {
// Code block to be executed
}
Where:
initialization
: Initializes the loop control variable (executed only once before the loop starts).condition
: Checked before each iteration; iftrue
, the loop continues; iffalse
, the loop stops.update
: Updates the loop control variable after each iteration.
Simple Example:
public class ForLoopExample {
public static void main(String[] args) {
for (int i = 1; i <= 5; i++) {
System.out.println("Iteration: " + i);
}
}
}
Output:
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Iteration: 5
In this example, the loop starts with i = 1
, checks the condition i <= 5
, and increments i
by 1
after each iteration until the condition becomes false.
for
Loop
Variations of the Using Multiple Control Variables
You can declare multiple control variables in the for
loop, as long as they have the same data type.
public class MultiVariableForLoop {
public static void main(String[] args) {
for (int i = 1, j = 10; i <= 5; i++, j--) {
System.out.println("i = " + i + ", j = " + j);
}
}
}
Output:
i = 1, j = 10
i = 2, j = 9
i = 3, j = 8
i = 4, j = 7
i = 5, j = 6
Nested Loops
A nested loop is a loop inside another loop. The inner loop runs completely for each iteration of the outer loop.
Example: Printing the Multiplication Table Using the for
Loop
public class MultiplicationTable {
public static void main(String[] args) {
for (int i = 2; i <= 9; i++) { // Outer loop: Iterates through tables from 2 to 9
System.out.println("Multiplication Table of " + i + ":");
for (int j = 1; j <= 10; j++) { // Inner loop: Multiplies from 1 to 10
System.out.println(i + " x " + j + " = " + (i * j));
}
System.out.println(); // Adds a blank line between tables
}
}
}
Output:
Multiplication Table of 2:
2 x 1 = 2
2 x 2 = 4
...
2 x 10 = 20
Multiplication Table of 3:
3 x 1 = 3
3 x 2 = 6
...
3 x 10 = 30
Explanation:
- The outer loop (
i
) iterates through numbers from2
to9
, representing different multiplication tables. - The inner loop (
j
) runs from1
to10
, performing multiplication for each number. - Each multiplication table is printed separately with a blank line in between.
Nested loops are very useful when working with matrices, tables, or multi-level data processing!
Omitting One or More Components
You can omit the initialization, condition, or update in the for
loop.
Omitting Initialization
If the loop control variable is declared beforehand, you can skip the initialization part.
public class SkipInitialization {
public static void main(String[] args) {
int i = 1;
for (; i <= 5; i++) {
System.out.println("i = " + i);
}
}
}
Omitting the Update Step
You can update the loop control variable inside the loop body instead of in the update
section.
public class SkipUpdate {
public static void main(String[] args) {
for (int i = 1; i <= 5;) {
System.out.println("i = " + i);
i++; // Update inside the loop body
}
}
}
for
Loop
Infinite If you omit the condition, the loop will run indefinitely.
public class InfiniteLoop {
public static void main(String[] args) {
for (;;) {
System.out.println("Infinite loop!");
}
}
}
Note: You should include a stopping condition (e.g.,
break
) to prevent the loop from running forever.
for
Loop
Iterating Through an Array Using the The for
loop is commonly used to iterate through array elements.
public class ArrayIteration {
public static void main(String[] args) {
int[] numbers = {2, 4, 6, 8, 10};
for (int i = 0; i < numbers.length; i++) {
System.out.println("Element at index " + i + ": " + numbers[i]);
}
}
}
for-each
Loop (Enhanced For Loop)
The Java provides the for-each
loop, which simplifies iterating through arrays or collections without needing an index variable.
for-each
Loop
Syntax of the for (DataType variable : collection) {
// Code block to be executed
}
Example:
public class ForEachExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
System.out.println("Value: " + num);
}
}
}
Output:
Value: 1
Value: 2
Value: 3
Value: 4
Value: 5
- Advantages of
for-each
: Clean, easy to read, and avoids index-related errors. - Disadvantages: You cannot access the index of the current element.
for
with break
and continue
Using break
to Exit the Loop Early
Using public class BreakExample {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
if (i == 6) {
break; // Stops the loop when i == 6
}
System.out.println(i);
}
}
}
Output:
1
2
3
4
5
continue
to Skip an Iteration
Using public class ContinueExample {
public static void main(String[] args) {
for (int i = 1; i <= 5; i++) {
if (i == 3) {
continue; // Skips i == 3
}
System.out.println(i);
}
}
}
Output:
1
2
4
5
Conclusion
The for
loop is a powerful tool for repeating a block of code in a controlled manner. You can use various variations of the for
loop, combined with break
and continue
, to optimize your program.
Next Lesson: Lesson 22. How to Use the for-each Loop in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
