Lesson 4. How to Declare Variables in Java | Learn Basic Java Programming
- Published on
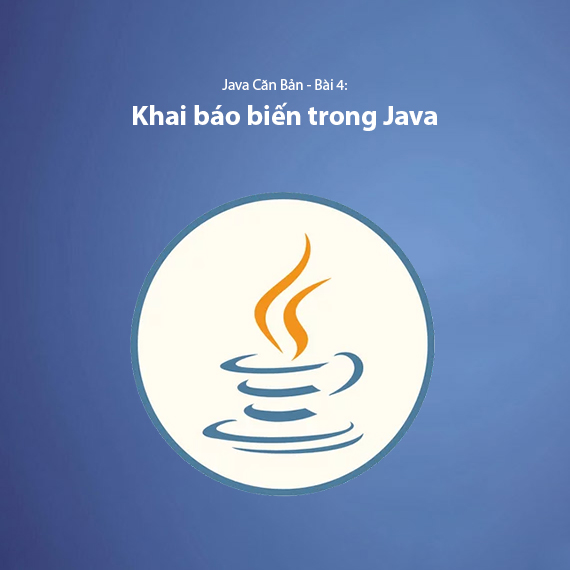
What is a Variable?
In Java programming, a variable is an essential part of every program. It stores values that the program can process, perform calculations on, or interact with users. Each variable in Java is declared with a specific data type to determine the kind of value it can store. This article will explain in detail how to declare variables in Java, the data types, and the rules to remember when working with variables.
Declaring Variables in Java
In Java, declaring a variable is an important step before using the variable. When declaring a variable, you need to specify:
- The data type of the variable: It defines the kind of value the variable can hold (integer, string, boolean, etc.).
- The variable name: The name of the variable for easy reference.
The basic structure of declaring a variable in Java is as follows:
dataType variableName;
- dataType: The data type of the variable (int, String, boolean, etc.).
- variableName: The name of the variable, following the naming rules in Java.
Example:
int age; // Declare variable age of type int (integer)
String name; // Declare variable name of type String (string)
boolean isStudent; // Declare variable isStudent of type boolean (true/false)
Declaring and Initializing Variables
When declaring a variable, you can also initialize it with a value immediately. This ensures the variable has an initial value when created.
Example:
int age = 25; // Declare and initialize variable age with the value 25
String name = "Alice"; // Declare and initialize variable name with the value "Alice"
boolean isStudent = true; // Declare and initialize variable isStudent with the value true
In this case, each variable is declared and initialized at the same time. This helps reduce errors from forgetting to initialize the variable before use.
Basic Data Types in Java
Java supports various data types for declaring variables, including primitive data types and reference data types. Below are some common data types that you will often encounter in Java.
Primitive Data Types
Primitive data types in Java are not objects and are used to store simple values. Below is a list of the most commonly used primitive data types:
Data Type | Size | Default Value | Value Range | Example |
---|---|---|---|---|
byte | 1 byte | 0 | -128 to 127 | byte b = 100; |
short | 2 bytes | 0 | -32,768 to 32,767 | short s = 32000; |
int | 4 bytes | 0 | -2,147,483,648 to 2,147,483,647 | int i = 1000; |
long | 8 bytes | 0L | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 | long l = 10000000000L; |
float | 4 bytes | 0.0f | ±3.40282347E+38F (7 digits precision) | float f = 3.14f; |
double | 8 bytes | 0.0d | ±1.79769313486231570E+308 (15 digits precision) | double d = 3.14159; |
char | 2 bytes | '\u0000' (null character) | 0 to 65,535 (Unicode values) | char c = 'A'; |
boolean | 1 bit | false | true or false | boolean b = true; |
Characteristics of Primitive Data Types:
- Fixed size: The size of these data types does not change regardless of the environment.
- High performance: Because they are managed directly in memory.
Reference Data Types
Reference data types in Java store references (addresses) to objects in memory instead of storing the actual values. These data types include:
- String: Stores a sequence of characters. Example:
"Hello World"
. - Array: Stores a collection of values of the same type. Example:
int[] numbers = {1, 2, 3, 4};
. - Class: Used to define objects. Example:
Person person = new Person();
. - Interface: Used to define methods that implementing classes must implement.
- Object Type: All classes in Java inherit from the
Object
class, which is the root class of all objects in Java.
Note: When declaring a value for the
char
data type, the value will be enclosed in single quotes ' ', for example: 'A'. Meanwhile, theString
data type will use double quotes " ", for example: "Hello".
Characteristics of Reference Data Types:
- Does not store the actual value: Instead, it stores the address of the object in memory.
- No fixed size: The size of a reference data type depends on the object it references.
Example:
String greeting = "Hello, World!";
int[] numbers = {1, 2, 3, 4, 5};
Variable Naming Rules
When declaring a variable, you must follow certain naming rules to make the variable easily identifiable and to avoid compilation errors. These rules include:
- Variable names must begin with a letter, underscore (_), or dollar sign ($).
- Subsequent characters can be letters, numbers, underscores, or dollar signs.
- Variable names cannot be the same as Java keywords (for example:
int
,class
,if
). - Variable names should follow the CamelCase convention (capitalize the first letter of each word after the first, for example:
myVariable
,totalAmount
). - Variable names cannot start with a number.
Correct Examples:
int myAge;
String userName;
double totalAmount;
Incorrect Examples:
int 1stValue; // Incorrect because variable name cannot start with a number
String class; // Incorrect because 'class' is a keyword in Java
Types of Variables in Java
Static Variables
A static variable is a variable declared with the static
keyword. Static variables do not belong to an object but to the class, meaning all objects of the class will share the same static variable.
Example:
class Counter {
static int count = 0; // Static variable
void increment() {
count++;
}
}
public class Main {
public static void main(String[] args) {
Counter obj1 = new Counter();
obj1.increment();
Counter obj2 = new Counter();
obj2.increment();
System.out.println("Count: " + Counter.count); // Count: 2
}
}
In the above example, the count
variable is static, so both obj1
and obj2
modify this variable. All objects of the Counter
class share the same count
variable.
Local Variables and Instance Variables
Local Variables
Local variables are variables declared within a method or a block of code. These variables only exist within the scope of the method or block and cannot be accessed from outside.
public void exampleMethod() {
int localVar = 10; // Local variable
System.out.println(localVar);
}
Instance Variables
Instance variables are variables declared within a class, but outside of any methods. They can be accessed from any method (except for static methods) in the class and have different values for each object of the class.
class Car {
String model; // Instance variable
void displayModel() {
System.out.println("Model: " + model);
}
}
Final Variables
When declaring a variable that you do not want to change its value after assignment, you can use the final
keyword. This variable becomes a final variable, meaning its value cannot be changed.
final int MAX_VALUE = 100;
When declaring a variable as final
, you must initialize it immediately, as its value will not change.
Conclusion
Declaring variables in Java is the first step in creating the data objects your program needs. Understanding the various data types, variable naming rules, and how to use local, instance, or static variables will help you program more efficiently. Once you grasp these concepts, you'll be able to build robust and maintainable Java programs.
Next article: Lesson 5. Data Types in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
