Lesson 5. Learn About Data Types in Java | Learn Java Basics
- Published on
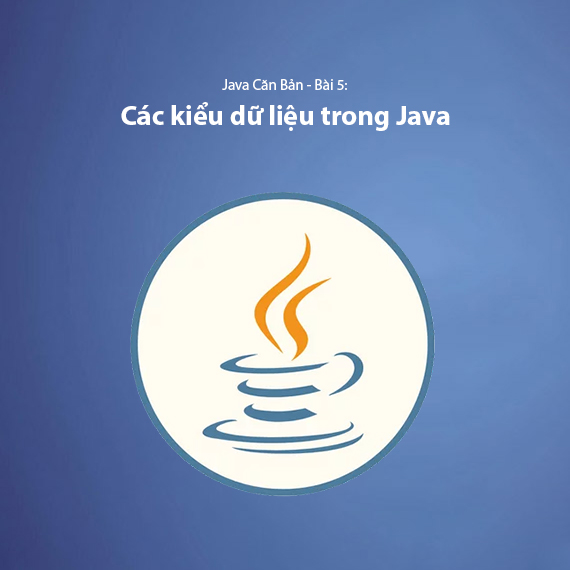
In Java, data types play an important role in declaring and determining the kind of values a variable can store. Understanding data types in Java helps you write more efficient code, optimize memory usage, and improve program performance. Java divides data types into two main groups: Primitive Data Types and Reference Data Types.
Primitive Data Types
Primitive data types in Java are the most basic types provided by the language. These data types are used to store values such as numbers, characters, etc. Java provides 8 primitive data types, each having a fixed size and a specific range of values.
Here is a summary table of the primitive data types in Java:
Data Type | Size | Value Range |
---|---|---|
byte | 1 byte | -128 to 127 |
short | 2 bytes | -32,768 to 32,767 |
int | 4 bytes | -2,147,483,648 to 2,147,483,647 |
long | 8 bytes | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | 4 bytes | 3.4e−038 to 3.4e+038 |
double | 8 bytes | 1.7e−308 to 1.7e+308 |
char | 2 bytes | 0 to 65,535 (stores Unicode characters) |
boolean | 1 bit | true or false |
Characteristics of Primitive Data Types:
- Fixed Size: The size of these data types does not change, regardless of the execution environment.
- High Performance: Since they are stored directly in memory and do not require complex management.
For example, declaring some variables with primitive data types in Java:
int number = 100;
float pi = 3.14f;
char grade = 'A';
boolean isValid = true;
Reference Data Types
Unlike primitive data types, reference data types in Java do not store the actual value but store a reference address to the object in memory (the actual object is stored in the heap memory).
These reference data types can store multiple values at once and can change size depending on the data being stored.
Reference Data Types in Java include:
-
String: Stores a sequence of characters.
String name = "John Doe";
-
Array: Stores a collection of values of the same type.
int[] numbers = {1, 2, 3, 4, 5};
-
Class: Used to define objects. A class is a basic reference data type in Java, and all objects in Java are created from a class.
class Person { String name; int age; }
-
Interface: Stores methods that a class must implement.
interface Animal { void sound(); }
-
Object: All classes in Java inherit from the
Object
class. Each object is an instance of a class.
Characteristics of Reference Data Types:
- Does not store the value directly: Reference data types store the address of the object in memory.
- No fixed size: The size of reference objects depends on the type of object and the number of components inside.
Example of declaring an object with a reference data type:
Person person = new Person();
person.name = "Alice";
person.age = 25;
Comparison Between Primitive and Reference Data Types
Characteristic | Primitive Data Types | Reference Data Types |
---|---|---|
Stores Value | Stores the value directly | Stores the memory address of the object |
Size | Fixed | Varies depending on the object |
Performance | High | Lower due to dynamic memory management |
Examples | int, char, boolean | String, Array, Object |
var
Data Type (From Java 10 onwards)
Java 10 introduced the var
data type, which allows you to declare variables without explicitly specifying their data type. When using var
, Java will automatically infer the data type based on the value assigned to the variable.
This helps make the code shorter and more readable, especially when working with complex data types or when you don’t need to worry about the specific data type. However, it’s important to note that the var
type can only be used when the variable is immediately initialized with a value.
Example:
var number = 10; // Java infers that number is of type int
var name = "Java"; // Java infers that name is of type String
Although var
reduces the need to declare the data type, you still need to ensure that the value assigned can clearly infer its type. Otherwise, you will encounter a compilation error.
Note:
var
can only be used with local variables within a method, and cannot be used for global variables or variables in classes.
Summary
- Primitive data types in Java are basic types used to store simple values such as integers, floating-point numbers, characters, and boolean values. They have a fixed size and high performance.
- Reference data types are used to store objects and arrays. They store the address of the object rather than the actual value and do not have a fixed size.
Understanding data types in Java helps you choose the correct type for each situation, optimize memory usage, and write more efficient code.
Next Lesson: Lesson 6. Constants in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
