Lesson 6. Understanding Constants in Java | Learn Java Basics
- Published on
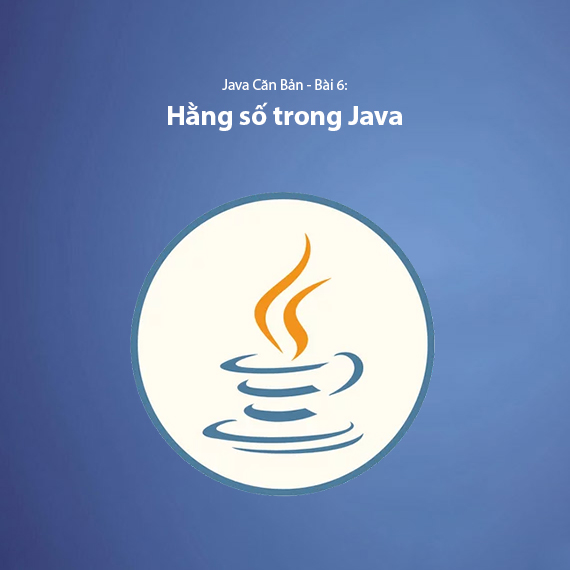
- What are Constants in Java?
- How to Declare Constants in Java
- Examples of Constants
- Constant Naming Conventions
- Constants in Classes and Objects
- Class Constants (Static Final)
- Instance Constants (Final)
- Benefits of Using Constants in Java
- Examples of Constants in Java
- Constants for Defining Limits
- Constants for Defining Mathematical Values
- Conclusion
What are Constants in Java?
In Java programming, constants are values that do not change throughout the execution of a program. When you need to use a fixed value that should not be altered, you can use constants. Constants make the program more understandable and prevent errors caused by unintended value changes.
Java provides the final
keyword to declare constants. Once you assign a value to a variable using final
, you cannot modify that value afterward.
How to Declare Constants in Java
To declare a constant in Java, use the final
keyword. The general syntax is:
final <data_type> <constant_name> = <value>;
final
: Keyword used to declare a constant.<data_type>
: The data type of the constant (e.g.,int
,double
,String
, etc.).<constant_name>
: The name of the constant, usually written in uppercase following Java naming conventions.<value>
: The value assigned to the constant, which cannot be changed.
Examples of Constants
Here are some examples of constant declarations in Java:
public class ConstantsExample {
public static final int MAX_USERS = 100; // Integer constant
public static final double PI = 3.14159; // Double constant
public static final String GREETING = "Hello!"; // String constant
public static void main(String[] args) {
System.out.println("Max Users: " + MAX_USERS);
System.out.println("Pi: " + PI);
System.out.println("Greeting: " + GREETING);
}
}
When running the above program, the output will be:
Max Users: 100
Pi: 3.14159
Greeting: Hello!
Constant Naming Conventions
- Constant names in Java are typically written in uppercase letters, with words separated by underscores (
_
).
Examples:
MAX_USERS
PI
EARTH_GRAVITY
This convention makes it easy to distinguish between regular variables and constants in your code.
Constants in Classes and Objects
When declaring constants in a class, you can choose to declare them as class constants (static final) or instance constants (final).
Class Constants (Static Final)
If a constant is shared among all objects of a class, declare it as static final
:
public class MathConstants {
public static final double PI = 3.14159;
public static void main(String[] args) {
System.out.println("Pi: " + MathConstants.PI);
}
}
Instance Constants (Final)
If you want a constant to belong to each instance of a class, declare it as final
(without static
):
public class Person {
public final String name;
public Person(String name) {
this.name = name; // Assigning value to the constant during object creation
}
public static void main(String[] args) {
Person person1 = new Person("John");
System.out.println(person1.name); // John
}
}
Benefits of Using Constants in Java
-
Prevents unintended value changes: When you declare a constant, you ensure that its value remains unchanged throughout the program execution, preventing errors caused by accidental modifications.
-
Enhances clarity and readability: Constants make the source code more readable because their names often have clear meanings. Using constants instead of "hard-coded" values helps you understand their purpose more easily.
-
Easier code maintenance: If you need to update a constant value, you only have to change it in one place (where it's declared), rather than searching for and modifying multiple occurrences in the source code.
-
Performance optimization: The Java compiler can optimize constant usage since their values do not change.
Examples of Constants in Java
Constants for Defining Limits
Suppose you need to define some limits in your application, such as the maximum number of users or login attempts.
public class AppConfig {
public static final int MAX_LOGIN_ATTEMPTS = 5;
public static final int MAX_USERS = 1000;
public static void main(String[] args) {
System.out.println("Max Login Attempts: " + MAX_LOGIN_ATTEMPTS);
System.out.println("Max Users: " + MAX_USERS);
}
}
Constants for Defining Mathematical Values
When working with fixed mathematical values such as Pi or Euler's number, you can declare them as constants:
public class MathConstants {
public static final double PI = 3.14159;
public static final double E = 2.71828;
public static void main(String[] args) {
System.out.println("Pi: " + PI);
System.out.println("Euler's number: " + E);
}
}
Conclusion
- Constants in Java are values that cannot be changed after being assigned.
- To declare a constant in Java, use the
final
keyword. - Constants can be declared with primitive data types (
int
,double
,char
) or reference data types (String
,Array
, etc.). - Constants improve code readability, prevent unintended value changes, and enhance program performance.
Next lesson: Lesson 7. How to Write Comments in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
