Lesson 13. Data Assignment in Java | Learn Java Basics
- Published on
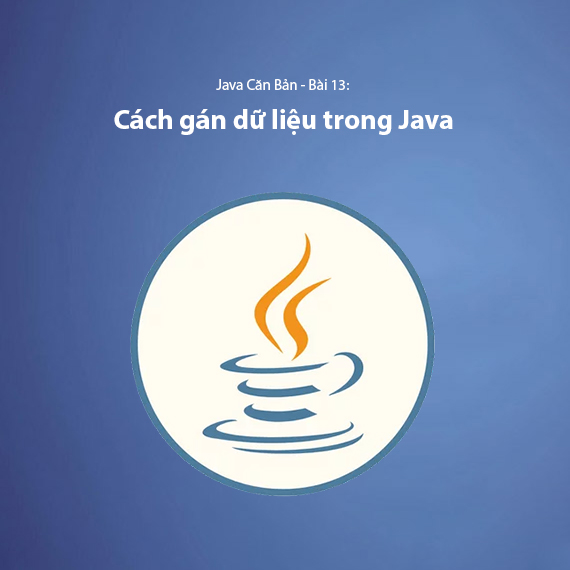
- What is Data Assignment in Java?
- Assigning Values to Variables
- Direct Value Assignment
- Assigning Values Using Expressions
- Assigning Values to Arrays
- Assigning Data to Objects
- Assigning Data Using Attributes
- Assigning Data Using a Constructor
- Assigning Values Using Setter Methods
- Assigning Data Using Collections
- Assigning Data Using Arrays.fill()
- Conclusion
What is Data Assignment in Java?
In Java, "data assignment" is the action of storing a value into a variable using assignment operators (=
). In simple terms, when you want to store some information in memory for later use, you need to assign a value to a variable. Java supports many ways of assigning data depending on the data type and data structure you use.
Assigning Values to Variables
Direct Value Assignment
You can assign a value directly to a variable using the =
operator.
int a = 10; // Variable a is assigned the value 10
double b = 3.14; // Variable b holds the floating point number 3.14
char c = 'A'; // Variable c holds the character 'A'
String str = "Hello, Java!"; // Variable str holds the string "Hello, Java!"
Assigning Values Using Expressions
In addition to direct assignment, you can assign a value to a variable using a mathematical or logical expression.
int x = 5, y = 10;
int sum = x + y; // sum = 15 (Sum of x and y)
double average = (x + y) / 2.0; // average = 7.5 (Average of x and y)
Assigning Values to Arrays
An array is a collection of multiple values of the same type. When declaring an array, you can assign values to it immediately or use the new
keyword.
int[] numbers = {1, 2, 3, 4, 5}; // Integer array
String[] names = new String[]{"Alice", "Bob", "Charlie"}; // String array
Assigning Data to Objects
Assigning Data Using Attributes
You can assign a value directly to an attribute of an object after creating that object.
class Person {
String name;
int age;
}
Person p = new Person();
p.name = "John";
p.age = 25; // Object p has name "John" and age 25
Assigning Data Using a Constructor
A better way to assign data to an object is by using a constructor (initialization method).
class Person {
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
}
Person p = new Person("Alice", 30); // Create object with name "Alice" and age 30
Assigning Values Using Setter Methods
In object-oriented programming, instead of assigning values directly, you can use a setter
method to ensure encapsulation.
class Person {
private String name;
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Person p = new Person();
p.setName("Bob");
System.out.println(p.getName()); // Prints "Bob"
Assigning Data Using Collections
Collections like List
and Map
help store lists and key-value pairs flexibly.
import java.util.*;
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
Map<String, Integer> map = new HashMap<>();
map.put("Alice", 25);
map.put("Bob", 30);
list.add("Apple")
: Adds the element "Apple" to the list.map.put("Alice", 25)
: Assigns the value 25 to the key "Alice" in the HashMap.
Assigning Data Using Arrays.fill()
Arrays.fill()
helps quickly assign a value to all elements of an array.
import java.util.Arrays;
int[] arr = new int[5];
Arrays.fill(arr, 100); // Array arr will contain {100, 100, 100, 100, 100}
Instead of assigning each element of the array individually, you can use Arrays.fill()
to assign the same value to all elements.
Conclusion
Data assignment in Java is an essential part of programming. Java provides many ways to assign values, making it flexible to work with data. You can assign values directly, use expressions, manipulate objects, arrays, collections, or take advantage of helper methods like Arrays.fill()
. Understanding data assignment will help you write more efficient code and optimize your programs.
Next Article: Article 14. Comparison Operators and Logical Operators in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
