Article 14. Comparison and Logical Operators in Java | Learn Basic Java
- Published on
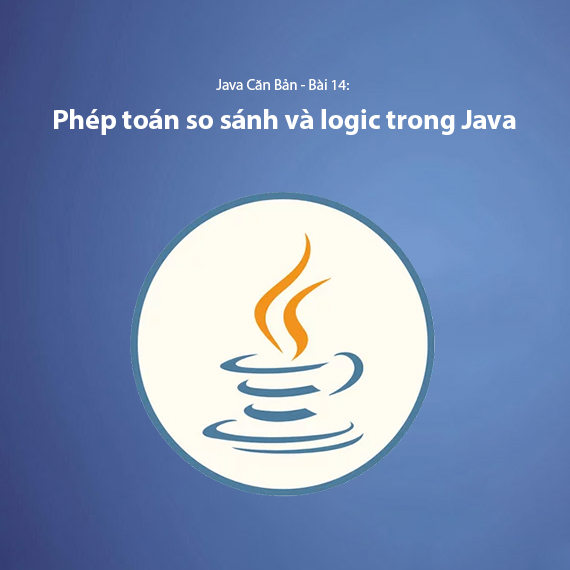
In Java programming, comparison and logical operators are crucial tools that allow you to check conditions and make decisions during the program execution. By using these operators, you can compare values, combine conditions, and evaluate them to control the flow of the program. Below is a detailed overview of comparison and logical operators in Java.
Comparison Operators
Comparison operators in Java are used to compare values and return a boolean value (true
or false
) based on the result of the comparison. These operators are essential for performing conditional statements such as if
, else if
, or loops.
Here are the basic comparison operators:
-
==
(Equal to): Checks if two values are equal. This operator compares the value of two objects or variables and returnstrue
if they are equal, otherwisefalse
.Example:
int a = 5, b = 5; boolean result = a == b; // true, because 5 == 5
Note: For objects (e.g.,
String
),==
checks if two objects refer to the same memory location, not their content. To compare the content of strings, you should use the.equals()
method. -
!=
(Not equal to): Checks if two values are not equal. If they are different, the result will betrue
, otherwisefalse
.Example:
int a = 5, b = 10; boolean result = a != b; // true, because 5 != 10
-
>
(Greater than): Checks if the value on the left is greater than the value on the right. If true, the result istrue
.Example:
int a = 10, b = 5; boolean result = a > b; // true, because 10 > 5
-
<
(Less than): Checks if the value on the left is less than the value on the right.Example:
int a = 5, b = 10; boolean result = a < b; // true, because 5 < 10
-
>=
(Greater than or equal to): Checks if the value on the left is greater than or equal to the value on the right. The result istrue
if the condition is satisfied.Example:
int a = 10, b = 10; boolean result = a >= b; // true, because 10 >= 10
-
<=
(Less than or equal to): Checks if the value on the left is less than or equal to the value on the right.Example:
int a = 5, b = 10; boolean result = a <= b; // true, because 5 <= 10
Logical Operators
Logical operators in Java help combine multiple conditions and form complex logical expressions. They return a boolean value (true
or false
) based on the evaluation of these operators.
Here are the basic logical operators:
-
&&
(AND logic): Used when you want to check if all conditions are true. This operator returnstrue
only if all conditions are true.Example:
int a = 5, b = 10; boolean result = (a > 0) && (b > 5); // true, because both conditions are true
Explanation: In the example above, we check if
a
is greater than 0 andb
is greater than 5. Since both conditions are true, the result istrue
. -
||
(OR logic): Used to check if at least one of the conditions is true. This operator returnstrue
if at least one of the conditions is true.Example:
int a = 5, b = 2; boolean result = (a > 0) || (b > 5); // true, because a > 0 is true
Explanation: Only one condition needs to be true. In this case,
a > 0
is true, so the result will betrue
. -
!
(NOT logic): Used to negate a boolean value. If the value istrue
, the result will befalse
, and vice versa.Example:
boolean isTrue = false; boolean result = !isTrue; // true, because !false is true
Explanation: In the example above, since
isTrue
isfalse
, using!isTrue
will returntrue
, and theif
statement will be executed.
How to Use Comparison and Logical Operators
Comparison and logical operators are commonly used in conditional statements (if
, else
, switch
, etc.) and loops (for
, while
, etc.).
if
statement:
Example using an int a = 5, b = 10;
if (a > 0 && b > 5) {
System.out.println("Both a and b satisfy the condition");
}
Explanation: In the example above, we use the &&
operator to check if both conditions (a > 0)
and (b > 5)
are true. If both conditions are true, the statement inside the if
will be executed.
else
statement:
Example using an int a = 5, b = 10;
if (a > 0 || b < 5) {
System.out.println("At least one condition is true");
} else {
System.out.println("Both conditions are false");
}
Explanation: The ||
operator checks if at least one condition is true. In this case, a > 0
is true, so the if
statement will be executed.
!
operator:
Example using the boolean isActive = false;
if (!isActive) {
System.out.println("The object is not activated");
}
Explanation: The !
operator negates the boolean value of isActive
. Since isActive
is false
, using !isActive
will return true
, and the if
statement will be executed.
Things to Remember When Using Comparison and Logical Operators
-
Note when using
==
with objects: For primitive types (such asint
,float
,double
, etc.), the==
operator compares values directly. However, for objects (likeString
,ArrayList
, etc.),==
only compares memory addresses, not the values inside. To compare the content of objects, you should use the.equals()
method. -
Combining logical operators: You can combine multiple logical operators in one expression, but you need to pay attention to the precedence of the operators to avoid incorrect results. The
&&
and||
operators have lower precedence than comparison operators (>
,<
,==
, etc.).
Conclusion
Comparison and logical operators in Java are powerful tools that help developers check conditions and make decisions during programming. Understanding how to use them will help you write clean, understandable, and efficient code. Be sure to combine them with conditional statements and loops to make your program more flexible and intelligent!
Next article: Article 15. Conditional Operators in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
