Lesson 15. Conditional Operator in Java | Learn Java Basics
- Published on
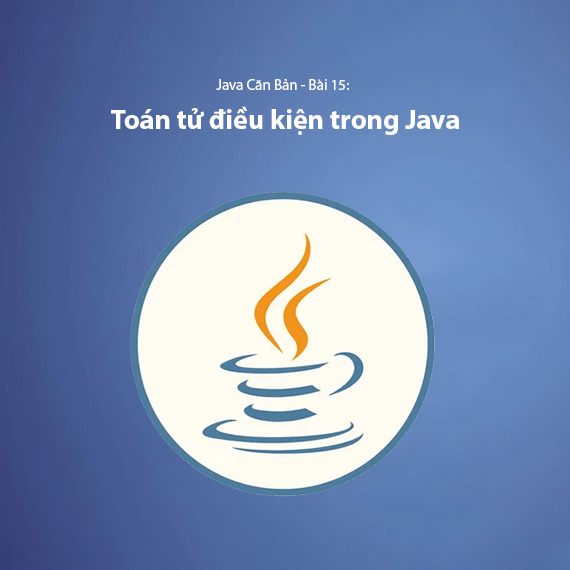
Conditional Operator in Java
In Java programming, the Conditional Operator (also known as the Ternary Operator) is a very useful tool that helps shorten if-else statements with a concise, easy-to-understand syntax. This operator is commonly used when you want to make a choice between two values based on a condition.
In this article, we will explore how to use the Conditional Operator in Java, its structure, and practical examples.
Structure of the Conditional Operator
The Conditional Operator in Java follows this syntax:
condition ? value_if_true : value_if_false;
- condition: The condition you want to evaluate. This condition can be a logical expression that returns either
true
orfalse
. - value_if_true: The value returned if the condition is
true
. - value_if_false: The value returned if the condition is
false
.
Example:
int a = 5;
int b = 10;
int max = (a > b) ? a : b;
System.out.println("The largest number is: " + max);
Explanation:
- If
a > b
(the condition is true), the value ofa
will be assigned tomax
. - If the condition is false (i.e.,
a <= b
), the value ofb
will be assigned tomax
.
The result will be:
The largest number is: 10
Practical Examples of Using the Conditional Operator in Java
The Conditional Operator is very helpful when you need to replace lengthy if-else
statements in simple cases. Below are some examples of how to use this operator.
Comparing Two Numbers
Suppose you want to compare two numbers and print the largest one. You can use the Conditional Operator instead of writing an if-else
structure.
int a = 7;
int b = 3;
int largest = (a > b) ? a : b;
System.out.println("The largest number is: " + largest);
Result:
The largest number is: 7
Checking Even or Odd
You can also use the Conditional Operator to check whether a number is even or odd.
int number = 8;
String result = (number % 2 == 0) ? "Even" : "Odd";
System.out.println(number + " is " + result);
Result:
8 is Even
Determining Grades
Suppose you want to determine a student's grade based on their score. You can use the Conditional Operator to do this.
int score = 85;
String grade = (score >= 90) ? "A" : (score >= 75) ? "B" : "C";
System.out.println("Grade: " + grade);
Result:
Grade: B
Here, the Conditional Operator is nested to check multiple conditions.
Benefits of Using the Conditional Operator
- Saves lines of code: By using the Conditional Operator, you can significantly reduce the number of lines in simple tasks, making your program shorter and easier to read.
- Increases readability: The syntax of the Conditional Operator is easy to understand and use, especially when you only need to check a simple condition.
- Convenient for complex expressions: When you need to perform multiple conditions in one expression, the Conditional Operator is a good choice, as you can nest them to form more complex conditions.
Limitations of the Conditional Operator
While the Conditional Operator is powerful and convenient, there are a few things you should keep in mind:
- Can be confusing: If you nest too many Conditional Operators, your code can become difficult to read and understand.
- Not suitable for complex conditions: For situations with many conditions or multiple actions, using
if-else
statements may be more appropriate, as the Conditional Operator's syntax can make the code harder to maintain.
Example of nesting too many Conditional Operators:
int a = 10;
int b = 5;
int c = 15;
int max = (a > b) ? ((a > c) ? a : c) : ((b > c) ? b : c);
System.out.println("The largest number is: " + max);
In this case, while the Conditional Operator is technically usable, the code becomes difficult to read and understand. Therefore, in more complex situations, using if-else
structures or separate methods will make the code easier to maintain.
Conclusion
The Conditional Operator in Java is a valuable tool that helps write concise and easy-to-understand code for simple condition checks. However, you should be cautious not to overuse the Conditional Operator, as too many nested operators can make the code harder to read and maintain.
With the examples and theory above, you now have a solid understanding of how to use the Conditional Operator in Java and apply it to real-world programming problems.
Next article: Article 16. Math Class and Mathematical Functions in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
