Lesson 16. Math Class and Mathematical Functions in Java | Learn Java Basics
- Published on
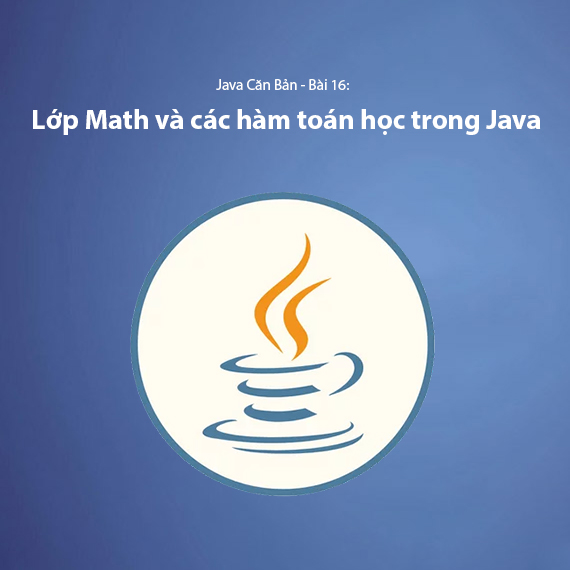
Introduction
In Java programming, mathematics plays a crucial role when handling complex calculations. To support these operations, Java provides a built-in class called Math. The Math class in Java offers many useful methods for arithmetic operations, ranging from basic calculations such as addition, subtraction, multiplication, and division to more complex operations like square roots, logarithms, and exponential functions.
In this article, we will explore the Math class in Java, the mathematical functions it provides, and how to use them in real-world problems.
Structure of the Math Class in Java
The Math class in Java belongs to the java.lang package, so you do not need to import it into your program. The Math class primarily provides static methods, meaning you can call them without creating an instance of the Math class.
General syntax of the Math class:
Math.method_name(parameters);
Below are some common methods of the Math class that you will frequently encounter in programming.
Basic Methods of the Math Class
-
Math.abs(double a)
Returns the absolute value of a number. This method is used to get the absolute value of a number, eliminating the negative sign (if any).Example:
double num = -10.5; double result = Math.abs(num); System.out.println(result); // Output: 10.5
-
Math.sqrt(double a)
Returns the square root of a number. This method is commonly used when you need to compute the square root.Example:
double num = 25; double result = Math.sqrt(num); System.out.println(result); // Output: 5.0
-
Math.pow(double a, double b)
Returns a raised to the power of b (a^b). This method is useful for exponentiation calculations.Example:
double base = 2; double exponent = 3; double result = Math.pow(base, exponent); System.out.println(result); // Output: 8.0
-
Math.max(double a, double b)
Returns the larger value between two numbers a and b.Example:
double num1 = 4.5; double num2 = 7.8; double result = Math.max(num1, num2); System.out.println(result); // Output: 7.8
-
Math.min(double a, double b)
Returns the smaller value between two numbers a and b.Example:
double num1 = 4.5; double num2 = 7.8; double result = Math.min(num1, num2); System.out.println(result); // Output: 4.5
-
Math.round(double a)
Rounds a number to the nearest integer.Example:
double num = 3.6; long result = Math.round(num); System.out.println(result); // Output: 4
Other Methods of the Math Class
-
Math.random()
Returns a random number between 0 (inclusive) and 1 (exclusive).Example:
double randomValue = Math.random(); System.out.println(randomValue); // Output: A random value in the range [0, 1)
-
Math.sin(double a)
Returns the sine of angle a (in radians).Example:
double angle = Math.PI / 2; // 90 degrees in radians double result = Math.sin(angle); System.out.println(result); // Output: 1.0
-
Math.cos(double a)
Returns the cosine of angle a (in radians).Example:
double angle = Math.PI; // 180 degrees in radians double result = Math.cos(angle); System.out.println(result); // Output: -1.0
-
Math.tan(double a)
Returns the tangent of angle a (in radians).Example:
double angle = Math.PI / 4; // 45 degrees in radians double result = Math.tan(angle); System.out.println(result); // Output: 1.0
-
Math.log(double a)
Returns the natural logarithm (base e) of a.Example:
double num = Math.E; // The mathematical constant e double result = Math.log(num); System.out.println(result); // Output: 1.0
-
Math.log10(double a)
Returns the base-10 logarithm of a.Example:
double num = 100; double result = Math.log10(num); System.out.println(result); // Output: 2.0
Real-World Applications of the Math Class in Java
- Solving Mathematical Equations
Suppose you want to find the roots of a quadratic equation:
double a = 1, b = -3, c = 2;
double discriminant = Math.pow(b, 2) - 4 * a * c;
if (discriminant > 0) {
double root1 = (-b + Math.sqrt(discriminant)) / (2 * a);
double root2 = (-b - Math.sqrt(discriminant)) / (2 * a);
System.out.println("Root 1: " + root1);
System.out.println("Root 2: " + root2);
} else {
System.out.println("The equation has no real roots.");
}
- Handling Angles and Measurements
You can use sin, cos, and tan functions to solve geometric problems involving angles.
double angle = Math.toRadians(30); // Convert 30 degrees to radians
double sine = Math.sin(angle);
System.out.println("Sin(30 degrees) = " + sine);
Conclusion
The Math class in Java is an extremely powerful and convenient tool for mathematical operations. By using the static methods provided by the Math class, you can perform calculations ranging from simple to complex, such as square roots, exponents, logarithms, arithmetic functions, and trigonometry, without needing to implement complex algorithms yourself.
With knowledge of the Math class, you can efficiently solve many mathematical problems in Java programming. Try applying these methods in your projects and experience the power that Java offers!
Next lesson: Lesson 17: If... Else Conditional Statements in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
