Lesson 7. Guide to Writing Comments in Java | Learn Java for Beginners
- Published on
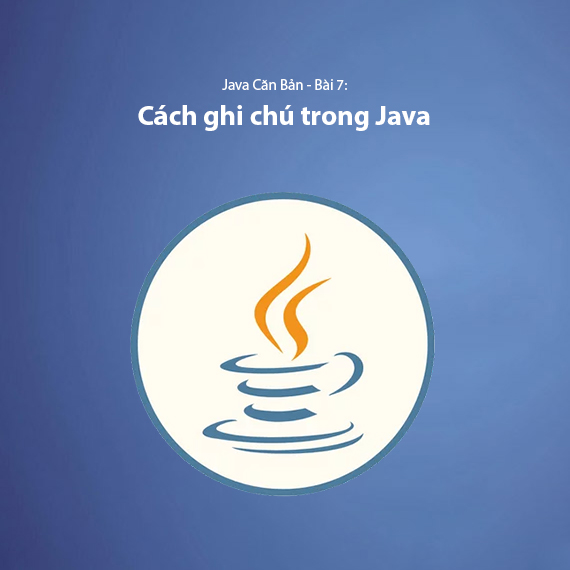
What are Comments in Java?
Comments in Java are blocks of code that help programmers explain their code without affecting the compilation or execution process. Using comments correctly makes the code easier to understand, supports documentation, and makes it easier to maintain the program.
Types of Comments in Java
Java supports three main types of comments:
- Single-line comments (
//
) - Multi-line comments (
/* ... */
) - Javadoc comments (
/** ... */
)
Single-line Comments
A single-line comment in Java starts with //
and applies to the rest of the line. Java will ignore the content after //
during compilation.
Example:
public class Main {
public static void main(String[] args) {
// Print the line "Hello, Java!"
System.out.println("Hello, Java!"); // This is also a comment
}
}
When to use single-line comments?
- To quickly comment on a specific line of code.
- To temporarily disable a line of code without deleting it.
Multi-line Comments
A multi-line comment in Java starts with /*
and ends with */
. This type can span multiple lines.
Example:
public class Main {
public static void main(String[] args) {
/*
* This is a multi-line comment.
* It can span multiple lines.
* It is commonly used to explain complex code.
*/
System.out.println("Hello, Java!");
}
}
When to use multi-line comments?
- To provide detailed explanations for important pieces of code.
- To disable multiple lines of code quickly.
Javadoc Comments
Javadoc is a special type of comment in Java, starting with /**
and ending with */
. It is used to create API documentation for classes, methods, or variables.
Example:
/**
* The Calculator class performs basic arithmetic operations.
*/
public class Calculator {
/**
* Adds two numbers.
*
* @param a The first number
* @param b The second number
* @return The sum of a and b
*/
public int add(int a, int b) {
return a + b;
}
}
When to use Javadoc?
- When you need to create documentation for Java code.
- When writing libraries or APIs for others to use.
You can generate HTML documentation from Javadoc by using the following command:
javadoc -d docs MyClass.java
Or in Eclipse, select Project > Generate Javadoc to automatically generate the documentation.
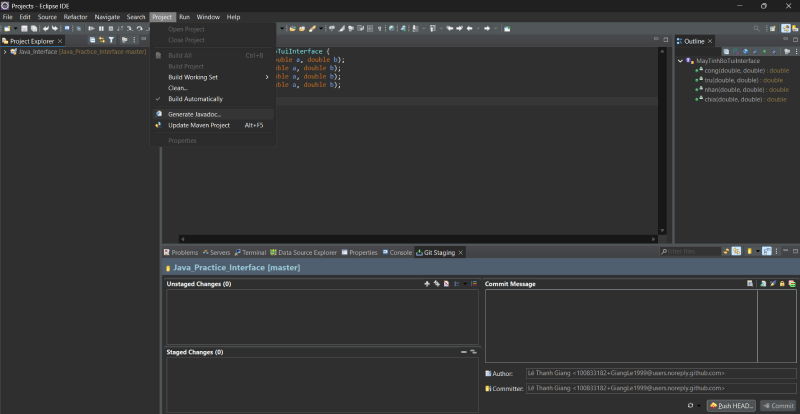
Important Javadoc Tags
Javadoc supports several special tags that help document your code in more detail.
Tag | Function |
---|---|
@param | Describes the input parameter of a method |
@return | Describes the return value of a method |
@author | The author of the source code |
@version | The version of the source code |
@see | Links to another class/method |
@deprecated | Marks a method/class that should no longer be used |
Example Using Multiple Javadoc Tags:
/**
* The MathUtils class contains utility methods for mathematical operations.
* @author John Doe
* @version 1.0
*/
public class MathUtils {
/**
* Calculates the factorial of a positive integer.
*
* @param n The positive integer
* @return The factorial of n
* @throws IllegalArgumentException if n < 0
*/
public static int factorial(int n) {
if (n < 0) {
throw new IllegalArgumentException("n must be >= 0");
}
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i;
}
return result;
}
}
Conclusion
- Comments help make code easier to understand, especially when working in teams.
- Use the right type of comment for the right purpose:
//
for short comments./* ... */
for long comments or disabling multiple lines./** ... */
to create API documentation with Javadoc.
- Don’t overuse comments. Only comment when necessary to avoid cluttering your code.
Next lesson: Lesson 8. How to Check and Handle Compilation Errors in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
