Lesson 8. Guide to Checking and Handling Compilation Errors in Java | Learn Java Basics
- Published on
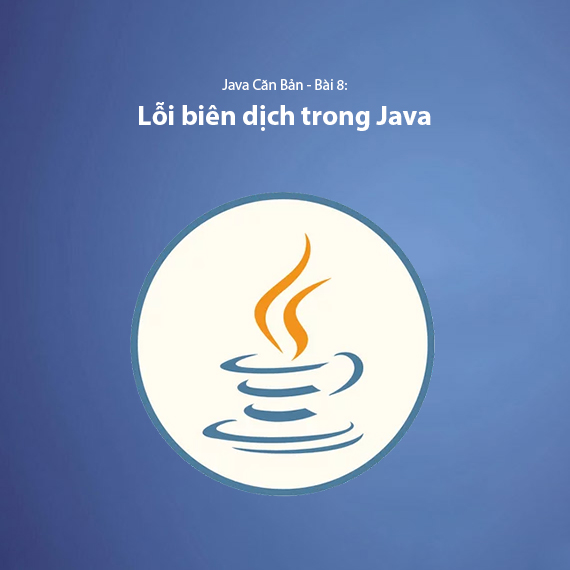
- What are Compilation Errors in Java?
- Types of Compilation Errors in Java
- Syntax Errors
- Undeclared Variables/Methods
- Data Type Errors
- Missing Libraries
- How to Check Compilation Errors
- Using the Java Compiler (javac)
- Using an IDE (Eclipse, IntelliJ, NetBeans)
- How to Handle Compilation Errors
- Read and Understand the Error Messages
- Fix Syntax Errors
- Check Variables and Methods
- Check Data Types
- Add Libraries and Correct Imports
- Checking and Handling Runtime Errors
- Conclusion
What are Compilation Errors in Java?
Compilation errors in Java are errors that occur when your Java program cannot be compiled into machine code that Java can understand and execute. This can happen for various reasons, such as incorrect syntax, missing classes, missing parentheses, or other issues related to data types. Handling compilation errors is an essential part of the programming process, helping you write clean, syntactically correct, and maintainable code.
In this article, we will learn how to check and handle compilation errors in Java.
Types of Compilation Errors in Java
Syntax Errors
Syntax errors are the most common errors and occur when you write code that does not follow the syntax rules of the Java language. These errors may include:
- Missing semicolon (
;
) at the end of a line. - Using parentheses incorrectly.
- Incorrect placement of keywords (such as
public
,static
,void
). - Invalid variable or class names.
Example:
public class Main {
public static void main(String[] args) {
System.out.println("Hello, Java!") // Error: missing semicolon
}
}
Undeclared Variables/Methods
This error occurs when you try to use a variable or method that has not been declared.
Example:
public class Main {
public static void main(String[] args) {
int x = 5;
System.out.println(y); // Error: variable y is not declared
}
}
Data Type Errors
Java is a statically-typed programming language, meaning variables must be declared with a specific data type. Data type errors occur when you try to assign an invalid value to a variable.
Example:
public class Main {
public static void main(String[] args) {
int num = "Hello"; // Error: incompatible data types
}
}
Missing Libraries
When you use external libraries in your Java program, if the library is not added to the classpath or you haven't imported it correctly, you'll encounter a compilation error.
Example:
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in); // Error: missing import java.util.Scanner
}
}
How to Check Compilation Errors
Using the Java Compiler (javac)
Java provides a command-line tool called javac
to compile .java
files into .class
bytecode files. When using javac
, if your code contains compilation errors, the tool will display detailed error messages.
How to use it:
- Open the terminal (or Command Prompt) and navigate to the directory containing the
.java
file. - Type the following command to compile:
javac Main.java
- If there are errors,
javac
will display an error message with the line of code that contains the error and a description of the problem.
Example error message:
Main.java:3: error: ';' expected
System.out.println("Hello, Java!")
^
1 error
Using an IDE (Eclipse, IntelliJ, NetBeans)
If you're using an IDE (Integrated Development Environment) like Eclipse, IntelliJ IDEA, or NetBeans, these IDEs often provide automatic compilation error checking and display errors directly in the user interface.
- Eclipse: When you compile your code, Eclipse will display the errors in the "Problems" tab or underline the erroneous parts in your source code.
- IntelliJ IDEA: IntelliJ will display warnings and errors directly in the source code window or in the status bar.
- NetBeans: Similarly, NetBeans will report errors when you attempt to compile your code.
How to Handle Compilation Errors
Read and Understand the Error Messages
One of the most important steps in handling compilation errors is reading and understanding the error messages from the compiler. The error messages typically provide information about:
- The line of code with the error: Helps you pinpoint the exact location of the issue in your code.
- The description of the error: Helps you understand the specific error (e.g., "missing semicolon," "cannot find symbol," "incompatible types").
- Suggested fixes: Sometimes, the error message will provide hints on how to resolve the issue.
Fix Syntax Errors
- Add a semicolon (
;
) at the end of the line. - Check the parentheses (
{
,}
,()
) to make sure they are correctly opened and closed. - Correct the placement of keywords (like
public
,private
,static
, etc.).
Check Variables and Methods
- Declare variables before using them.
- Check the scope of variables to ensure they are accessible within the method or class.
- Ensure methods are correctly defined and not missing.
Check Data Types
- Check the compatibility between data types of variables and the values assigned.
- Convert data types if necessary, for example, use
String.valueOf()
orInteger.parseInt()
.
Add Libraries and Correct Imports
- Check for required libraries and ensure they are correctly imported.
- Ensure external libraries have been added to the classpath when compiling.
import java.util.Scanner; // Make sure the Scanner library is imported
Checking and Handling Runtime Errors
In addition to compilation errors, when running a Java program, you may encounter runtime errors (errors during execution), such as division by zero or accessing an array out of bounds. To handle runtime errors, you can use mechanisms like try-catch
to catch and handle exceptions.
Example of a runtime error:
public class Main {
public static void main(String[] args) {
try {
int result = 10 / 0; // Error: division by zero
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero!");
}
}
}
Conclusion
- Compilation errors are errors that you can easily check and fix through detailed error messages from the compiler or IDE.
- Fixing compilation errors often involves checking the syntax, declaring variables, and ensuring your source code follows Java's rules.
- Using error-checking tools like
javac
or IDEs helps you quickly identify and fix errors. - Handling runtime errors can be done by using exception mechanisms (
try-catch
).
Checking and handling compilation errors not only ensures your program runs correctly but also helps you become a more careful and professional programmer.
Next article: Lesson 9. How to Read Input from the Keyboard in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
