Lesson 20. Check the Number of Days in a Month with Switch ... Case | Learn Basic Java
- Published on
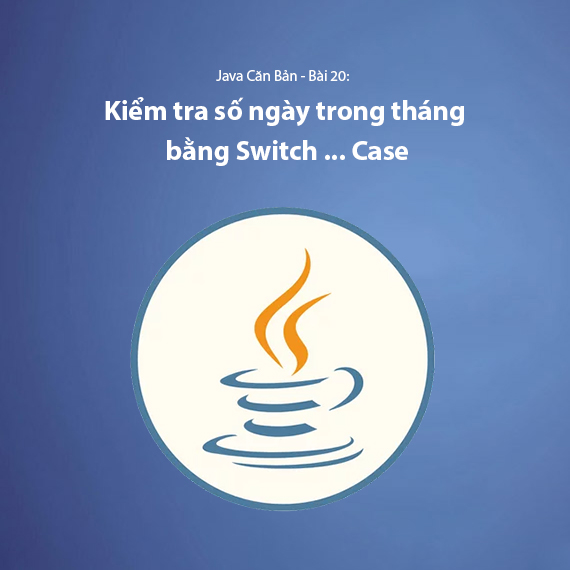
To solve the problem of checking the number of days in a month using the switch
statement in Java, before writing the code, you need to plan the steps clearly. Below are the detailed steps to solve this problem:
Steps to Solve the Problem
-
Determine the Input
- The user will input two values: the month (1-12) and the year (which could be a leap year or not).
- These values will be taken from the keyboard or the user interface.
-
Check the Validity of the Month
- The month must be in the range from 1 to 12.
- If the month value is invalid, an error message should be displayed, and the program should either terminate or ask the user to re-enter the value.
-
Handle Days in Fixed-Number Months
- The months with a fixed number of days are:
- January, March, May, July, August, October, and December have 31 days.
- April, June, September, and November have 30 days.
- Use the
switch
statement to handle these months.
- The months with a fixed number of days are:
-
Handle February
- February has a variable number of days depending on whether it's a leap year.
- You need to check if the year is a leap year:
- A leap year is one that is divisible by 4, but not by 100, or divisible by 400.
- If the year is a leap year, February has 29 days; otherwise, it has 28 days.
-
Display the Result
- After determining the number of days in the month, print the result for the user, including:
- The number of days in the given month and year.
-
Handle Errors (if any)
- If the user inputs an invalid month (not between 1 and 12), the program should notify the user and terminate or prompt for re-entry.
- If the entered year is invalid (such as a negative year), the program should handle it appropriately.
Writing the Code
Here is the Java code for the problem of checking the number of days in a month:
import java.util.Scanner;
public class DaysInMonth {
public static void main(String[] args) {
// Step 1: Input month and year
Scanner scanner = new Scanner(System.in);
System.out.print("Enter month (1-12): ");
int month = scanner.nextInt(); // Input month
System.out.print("Enter year: ");
int year = scanner.nextInt(); // Input year
// Step 2: Check the validity of the month
if (month < 1 || month > 12) {
System.out.println("Invalid month.");
return; // Exit the program if the month is invalid
}
// Step 3: Declare a variable to store the number of days in the month
int daysInMonth = 0;
// Step 4: Handle the number of days in the month using the switch statement
switch (month) {
case 1: // January
case 3: // March
case 5: // May
case 7: // July
case 8: // August
case 10: // October
case 12: // December
daysInMonth = 31;
break;
case 4: // April
case 6: // June
case 9: // September
case 11: // November
daysInMonth = 30;
break;
case 2: // February
// Check for leap year
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
daysInMonth = 29; // Leap year
} else {
daysInMonth = 28; // Non-leap year
}
break;
}
// Step 5: Display the result
System.out.println("The number of days in month " + month + " of year " + year + " is: " + daysInMonth);
}
}
Run and Check the Results
After implementing the code, the program will prompt you to input the month and year to check the number of days in the month. Below are some examples and results when running the program:
Example 1:
- Input:
Month = 2
,Year = 2024
- Result: "The number of days in month 2 of year 2024 is: 29"
- Explanation: Since 2024 is a leap year, February has 29 days.
Example 2:
- Input:
Month = 2
,Year = 2023
- Result: "The number of days in month 2 of year 2023 is: 28"
- Explanation: 2023 is not a leap year, so February has 28 days.
Example 3:
- Input:
Month = 4
,Year = 2023
- Result: "The number of days in month 4 of year 2023 is: 30"
- Explanation: April always has 30 days in every year.
Example 4:
- Input:
Month = 12
,Year = 2025
- Result: "The number of days in month 12 of year 2025 is: 31"
- Explanation: December always has 31 days.
Example 5:
- Input:
Month = 13
,Year = 2025
- Result: "Invalid month."
- Explanation: The month must be between 1 and 12, so month 13 is invalid.
Summary of Steps to Perform
- Input the month and year from the user.
- Check the validity of the month (must be between 1 and 12).
- Handle months with fixed days (31 or 30 days).
- Check February and determine the number of days based on whether it's a leap year.
- Print the result showing the number of days in the corresponding month.
- Notify the user of errors if an invalid month is entered or if there are other errors.
Now, you can easily apply these steps to write code for the problem of checking the number of days in a month using switch case
in Java!
Next post: Lesson 21. How to Use the For Loop in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
