Lesson 18. Solving Quadratic Equations with Java | Learn Java for Beginners
- Published on
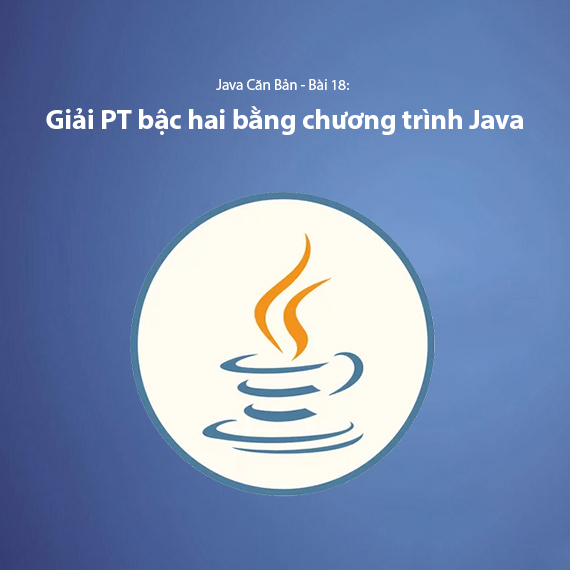
- What is a Quadratic Equation?
- Cases of a Quadratic Equation
- Writing a Program to Solve a Quadratic Equation in Java
- Code Explanation
- Running the Program and Checking Results
- Example 1: Equation with Two Distinct Solutions
- Example 2: Equation with One Repeated Solution
- Example 3: Equation with No Real Solutions
- Conclusion
What is a Quadratic Equation?
A quadratic equation is one of the basic types of mathematical equations that you commonly encounter. The equation is in the following form:
ax^2 + bx + c = 0
Where a
, b
, and c
are constants, and x
is the variable. The goal is to find the value of x
that satisfies this equation.
When solving a quadratic equation, we use the quadratic formula:
x = (-b ± √(b^2 - 4ac)) / 2a
Where:
b^2 - 4ac
is called thedelta
or the discriminant of the quadratic equation.- Based on the value of
delta
, we determine the number of solutions for the equation.
Cases of a Quadratic Equation
- If
delta
> 0: The equation has two distinct solutions. - If
delta
= 0: The equation has one repeated solution. - If
delta
< 0: The equation has no real solutions.
Writing a Program to Solve a Quadratic Equation in Java
In this lesson, we will write a Java program to solve a quadratic equation. This program will ask the user to input the values for a
, b
, and c
, then calculate and display the solution(s) if they exist. The steps are as follows:
- Step 1: Declare Variables and Input Data: We will need three variables
a
,b
, andc
to store the coefficients of the equation. Additionally, we will need a variable to store the value ofdelta
(discriminant). - Step 2: Calculate
delta
and Solutions: Use the formula above to calculate the value ofdelta
. Based on the value ofdelta
, we will determine the number of solutions and calculate the solutions of the equation. - Step 3: Display the Results: After the calculations, we will print the results to the screen, displaying the solution(s) of the equation.
From these steps, we proceed to write the code for the program as follows:
import java.util.Scanner;
public class SolveQuadraticEquation {
public static void main(String[] args) {
// Initialize Scanner object to receive user input
Scanner scanner = new Scanner(System.in);
// Input the coefficients of the quadratic equation
System.out.print("Enter coefficient a: ");
double a = scanner.nextDouble();
System.out.print("Enter coefficient b: ");
double b = scanner.nextDouble();
System.out.print("Enter coefficient c: ");
double c = scanner.nextDouble();
// Calculate delta
double delta = b * b - 4 * a * c;
// Handle cases based on the value of delta
if (a == 0) {
System.out.println("This is not a quadratic equation.");
} else if (delta > 0) {
// If delta > 0, the equation has two distinct solutions
double x1 = (-b + Math.sqrt(delta)) / (2 * a);
double x2 = (-b - Math.sqrt(delta)) / (2 * a);
System.out.println("The equation has two distinct solutions:");
System.out.println("Solution x1 = " + x1);
System.out.println("Solution x2 = " + x2);
} else if (delta == 0) {
// If delta = 0, the equation has one repeated solution
double x = -b / (2 * a);
System.out.println("The equation has one repeated solution: x = " + x);
} else {
// If delta < 0, the equation has no real solutions
System.out.println("The equation has no real solutions.");
}
// Close the scanner object
scanner.close();
}
}
Code Explanation
- Input Data: We use
Scanner
to get the coefficientsa
,b
, andc
from the user. - Calculate delta: The
delta
variable is calculated using the formulab^2 - 4ac
. - Handle the Cases:
- If
a
= 0, it's not a quadratic equation. - If
delta
> 0, the program calculates two distinct solutions. - If
delta
= 0, the program calculates one repeated solution. - If
delta
< 0, the program outputs that the equation has no real solutions.
- If
Running the Program and Checking Results
Example 1: Equation with Two Distinct Solutions
Input:
a
= 1b
= -5c
= 6
The program will calculate delta
= 1 and output:
The equation has two distinct solutions:
Solution x1 = 3.0
Solution x2 = 2.0
Example 2: Equation with One Repeated Solution
Input:
a
= 1b
= -4c
= 4
The program will calculate delta
= 0 and output:
The equation has one repeated solution: x = 2.0
Example 3: Equation with No Real Solutions
Input:
a
= 1b
= 2c
= 5
The program will calculate delta
= -16 and output:
The equation has no real solutions.
Conclusion
Solving quadratic equations in Java is a basic but important exercise that helps you understand how to use conditional statements (if-else
) and mathematical formulas in programming. The program above not only solves a mathematical problem but also helps you practice fundamental Java programming skills.
If you want to experiment further, you can extend this program to support solving quadratic equations with coefficients input from a file, or add a graphical user interface (GUI) to make the program more user-friendly.
Next lesson: Lesson 19. Switch ... Case Statements in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
