Lesson 9. A Detailed Guide on How to Read Input from the Keyboard in Java | Learn Basic Java
- Published on
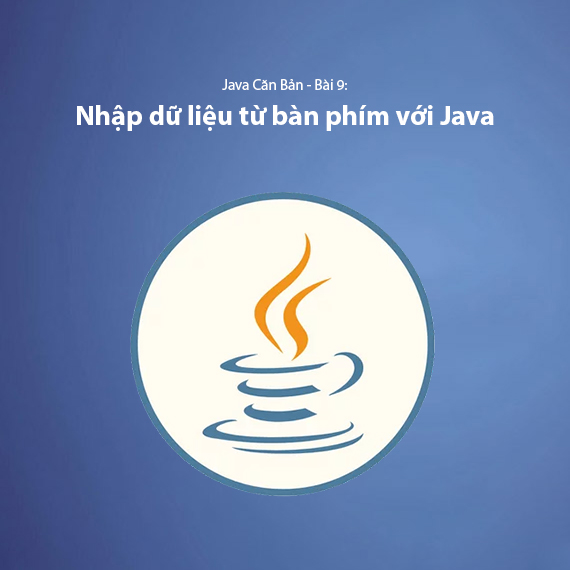
- Using Scanner (Most Common)
- Methods of Scanner:
- Issues When Using nextInt() or nextDouble() Before nextLine()
- Where Else Can Scanner Read Data From Besides the Keyboard?
- Using BufferedReader (Higher Performance)
- Using Console (Works Only in Terminal)
- When to Use Scanner, BufferedReader, or Console
- Use Scanner If:
- Use BufferedReader If:
- Use Console If:
- Conclusion
In Java, inputting data from the keyboard is a basic and essential step in the programming process. There are several ways to input data from the keyboard, but the most common methods are using the Scanner
, BufferedReader
, and Console
classes. Each method has its own advantages and usage depending on the program's requirements.
Scanner
(Most Common)
Using The Scanner
class, located in the java.util
package, helps you easily read data from the keyboard. This is the simplest and most accessible method for Java programmers.
Scanner
:
Methods of - Input an integer:
nextInt()
- Input a double:
nextDouble()
- Input a string:
next()
andnextLine()
- Input a character:
next().charAt(0)
Example:
import java.util.Scanner;
public class InputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Input an integer
System.out.print("Enter an integer: ");
int num = scanner.nextInt();
// Input a double
System.out.print("Enter a double: ");
double decimal = scanner.nextDouble();
// Input a character
System.out.print("Enter a character: ");
char ch = scanner.next().charAt(0);
// Clear the buffer before reading a string
scanner.nextLine();
// Input a string with spaces
System.out.print("Enter a string: ");
String str = scanner.nextLine();
// Display the results
System.out.println("\nYou entered:");
System.out.println("Integer: " + num);
System.out.println("Double: " + decimal);
System.out.println("Character: " + ch);
System.out.println("String: " + str);
scanner.close(); // Close the scanner to prevent resource leakage
}
}
Note:
next()
reads only one word, which does not contain spaces (a contiguous sequence of characters without spaces).nextLine()
reads the entire line, including spaces.- If using
nextInt()
ornextDouble()
beforenextLine()
, you need to callscanner.nextLine()
to clear the buffer after reading a number.
nextInt()
or nextDouble()
Before nextLine()
Issues When Using When you use nextInt()
or nextDouble()
before nextLine()
, you may encounter an issue where nextLine()
does not behave as expected because it will immediately pick up the newline character (\n
) left in the buffer. This causes the nextLine()
method to be skipped.
The newline character (
\n
) is the result of pressing the Enter key after entering a value. Each time you input data in Java, pressing Enter generates a newline character to mark the end of that line.
Example:
import java.util.Scanner;
public class ScannerProblem {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter age: ");
int age = scanner.nextInt(); // Reads the integer but does not consume the newline
System.out.print("Enter name: ");
String name = scanner.nextLine(); // Skipped because the `\n` is left in the buffer!
System.out.println("Age: " + age);
System.out.println("Name: " + name);
scanner.close();
}
}
Output:
Enter age: 25
Enter name: Age: 25
Name:
The program does not wait for the name input, and name
receives an empty string because the newline character was already consumed.
Where Else Can Scanner Read Data From Besides the Keyboard?
Although System.in
is the most common input source that Scanner
uses to read data from the keyboard, Scanner
can also read data from various other sources, including strings, files, or input streams from networks and systems. Below are examples demonstrating these different input sources:
Reading Data from a String
You can also use Scanner
to read data from a string. This is useful when you want to process an existing string instead of entering data directly from the keyboard.
Example:
import java.util.Scanner;
public class ScannerFromString {
public static void main(String[] args) {
String input = "Hello Java";
Scanner scanner = new Scanner(input); // Read data from the string
while (scanner.hasNext()) {
System.out.println(scanner.next()); // Read each word in the string
}
scanner.close();
}
}
Reading Data from a File
Scanner
can also read data from text files. You just need to pass a File
object to the constructor of Scanner
. This allows you to process data in the file without manually opening it.
Example:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class ScannerFromFile {
public static void main(String[] args) {
try {
File file = new File("data.txt"); // Create a File object for the file
Scanner scanner = new Scanner(file); // Read data from the file
while (scanner.hasNextLine()) {
System.out.println(scanner.nextLine()); // Read each line in the file
}
scanner.close();
} catch (FileNotFoundException e) {
System.out.println("File not found!");
}
}
}
Reading Data from an InputStream
Scanner
can also read data from input streams (InputStream), such as when reading data from a file or network. You can use FileInputStream
or other input streams to supply data to the Scanner
.
Example:
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Scanner;
public class ScannerFromInputStream {
public static void main(String[] args) {
try {
InputStream inputStream = new FileInputStream("data.txt"); // Create an input stream from the file
Scanner scanner = new Scanner(inputStream); // Read data from the input stream
while (scanner.hasNextLine()) {
System.out.println(scanner.nextLine()); // Read each line from the input stream
}
scanner.close();
} catch (IOException e) {
System.out.println("Error reading the file!");
}
}
}
BufferedReader
(Higher Performance)
Using The BufferedReader
class is another option that provides higher performance than Scanner
. However, you need to handle IOException
and convert data types when using it.
Example:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class BufferedReaderExample {
public static void main(String[] args) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
// Input a string
System.out.print("Enter a string: ");
String str = reader.readLine();
// Input an integer
System.out.print("Enter an integer: ");
int num = Integer.parseInt(reader.readLine());
// Input a double
System.out.print("Enter a double: ");
double decimal = Double.parseDouble(reader.readLine());
// Display results
System.out.println("\nYou entered:");
System.out.println("String: " + str);
System.out.println("Integer: " + num);
System.out.println("Double: " + decimal);
}
}
Note:
readLine()
returns a string, and you need to useInteger.parseInt()
orDouble.parseDouble()
to convert the data type.
Console
(Works Only in Terminal)
Using The Console
class from System
only works in a terminal environment and is not supported in IDEs like Eclipse or IntelliJ. Console
is useful when you need to input data securely, such as passwords.
Example:
import java.io.Console;
public class ConsoleExample {
public static void main(String[] args) {
Console console = System.console();
if (console == null) {
System.out.println("Console not supported in IDE!");
return;
}
// Input a string
String name = console.readLine("Enter your name: ");
// Input a password (hidden input)
char[] password = console.readPassword("Enter your password: ");
System.out.println("Hello, " + name);
}
}
Note:
System.console()
returnsnull
when running in an IDE.readPassword()
allows for password input without showing characters, enhancing security.
Scanner
, BufferedReader
, or Console
When to Use Scanner
If:
Use - You are writing an application that runs in an IDE (Eclipse, IntelliJ, NetBeans, VS Code, etc.).
- You need to input different types of data such as
int
,double
,char
,String
. - You need to input strings with spaces (use
nextLine()
). - You do not require high performance since
Scanner
can be slightly slower when handling large datasets.
BufferedReader
If:
Use - You are writing an application with high-performance requirements, especially when processing large amounts of data.
- You only need to read strings or integers/doubles and do not need to handle many complex data types.
- You want to work with input streams like reading files or network streams.
- You do not mind handling
IOException
and manually converting data types (such asInteger.parseInt()
orDouble.parseDouble()
).
Console
If:
Use - You are writing an application that runs in the terminal (command line) without the need for an IDE.
- You need to input passwords securely without showing characters, using
readPassword()
. - You do not need to read many numeric data types (since
Console
only reads strings; if you need numbers, you must convert them manually). - You do not require IDE support and want the application to run strictly in the command-line environment.
Conclusion
In Java, inputting data from the keyboard can be done through the Scanner
, BufferedReader
, and Console
classes.
Scanner
is the most common choice because it is easy to use and supports many types of data.BufferedReader
offers higher performance and is suitable for applications requiring fast processing.Console
provides good security but only works in terminal environments and is not supported in IDEs.
Choosing the appropriate input method depends on the specific requirements of your application and the development environment you are using.
Next lesson: Lesson 10. Data Type Conversion in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
