Lesson 3. Class in Java | Learn Basic Java
- Published on
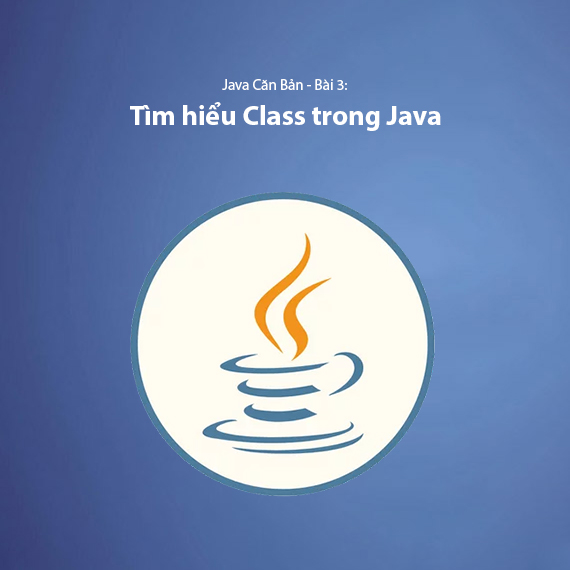
- What is a Class in Java?
- Basic Structure of a Class
- Simple Example of a Class
- Creating an Object from a Class
- Components of a Class
- Attributes (Fields)
- Methods
- Constructor
- Getter and Setter Methods
- Explanation of the Main Method in Java Class
- Types of Classes in Java
- Working with Eclipse
- How to Create a Java Class in a Project Using Eclipse IDE
- Quickly Create the main Method in a Class
- Run a Program in Eclipse
- Conclusion
What is a Class in Java?
A class in Java is a blueprint or template used to create objects. It defines the attributes (variables) and behaviors (methods) that objects of that class will have. In object-oriented programming (OOP), a class is a core component that helps organize and manage source code.
Basic Structure of a Class
A class in Java has the following basic structure:
class ClassName {
// Attributes of the class
dataType fieldName;
// Methods of the class
returnType methodName() {
// Method implementation
}
}
- ClassName: The name of the class (following the CamelCase rule).
- Field: The attributes or variables of the class.
- Method: The methods of the class (or behaviors of the object).
Simple Example of a Class
Here is a simple example of a class in Java:
class Person {
// Attributes of the class
String name;
int age;
// Method of the class
void introduce() {
System.out.println("Hi, my name is " + name + " and I am " + age + " years old.");
}
}
In the above example:
- Person is the class name.
- name and age are the attributes of the class.
- introduce() is a method that prints out an introduction about the object.
Creating an Object from a Class
Once you have defined a class, you can create objects from that class. This process is called object instantiation. To instantiate an object, you use the new keyword.
Example:
public class Main {
public static void main(String[] args) {
// Instantiate an object of class Person
Person person1 = new Person();
person1.name = "Alice";
person1.age = 25;
// Call the method of object person1
person1.introduce();
}
}
The result will be:
Hi, my name is Alice and I am 25 years old.
Components of a Class
Attributes (Fields)
Attributes of a class are variables that represent the data of an object. For example, in the Person class, name and age are attributes.
Methods
Methods in a class are actions that an object can perform. A method can change the values of attributes, perform operations, or print results to the screen.
Constructor
A constructor is a special method in a class used to initialize objects of the class. Constructors have the following main characteristics:
-
Same name as the class: The constructor always has the same name as the class it belongs to. This helps the JVM identify and call the constructor when creating an object.
-
No return type: This means that the constructor does not return any value, not even
void
. While methods in Java generally need to have a return type, constructors do not. -
Object initialization: Constructors are typically used to initialize the initial values for the object’s attributes. When an object is created, the constructor is automatically called to set up the initial state of the object.
-
Multiple constructors: A class can have multiple constructors with different parameters. This is known as constructor overloading. These constructors can initialize objects with different values depending on the program’s needs.
Example:
class Person {
String name;
int age;
// Constructor
Person(String name, int age) {
this.name = name;
this.age = age;
}
void introduce() {
System.out.println("Hi, my name is " + name + " and I am " + age + " years old.");
}
}
In this example, Person(String name, int age) is a constructor that helps initialize a Person object with values for name and age.
When creating a Person object, you can call the constructor like this:
public class Main {
public static void main(String[] args) {
Person person1 = new Person("Alice", 25);
person1.introduce();
}
}
Result:
Hi, my name is Alice and I am 25 years old.
Getter and Setter Methods
Getter and setter methods are used to access and modify the values of attributes in a class.
Example:
class Person {
private String name;
private int age;
// Getter and Setter for name
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
// Getter and Setter for age
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
With getter and setter methods, you can control the access and modification of class attributes from outside the class.
public class Main {
public static void main(String[] args) {
Person person1 = new Person();
person1.setName("Alice");
person1.setAge(25);
System.out.println(person1.getName());
System.out.println(person1.getAge());
}
}
Result:
Alice
25
Explanation of the Main Method in Java Class
The main method in Java is the entry point of the program. Every time you run a Java program, the Java Virtual Machine (JVM) will find and execute the main method from the specified class. The basic structure of the main method is:
public class Hello {
public static void main(String[] args) {
System.out.println("Hello");
}
}
In the example above, the main method accepts a parameter String[] args, which is an array of strings used to receive command-line arguments when the program is executed. This parameter allows you to pass data into the program when running it from the terminal or command line.
One important point is that the main method must be declared as static. This is because the main method is called without the need to create an object of the class. When a Java program is run, the JVM does not create an object of the class, it simply calls the main method. If it is not declared static, you would need to create an object of the class before calling the main method, which complicates the startup process and is not suitable for the role of the "starting point" of the program.
In more complex programs, the main method often does not contain much logic but only performs essential initialization steps. For example, in a large application, the main method might be used to:
- Create necessary objects (such as the main processing classes of the program).
- Set up necessary resources (such as database connections).
- Transfer control to other classes or objects to continue performing specific tasks.
The following example shows a main method in a more complex program, where it only starts the application and transfers control to another class:
public class Application {
public static void main(String[] args) {
System.out.println("Starting the program");
MyApp app = new MyApp();
app.run();
}
}
Here, the main method just starts the program, creates the MyApp object, and then calls the run method of the MyApp class to continue processing the specific tasks of the application.
A Java program can have many classes, but only one main method is needed to run. If there is no main method, the JVM will throw an error.
Types of Classes in Java
Java supports several types of classes, including:
- Normal Class: This is the most common type of class you’ve seen in previous examples.
- Abstract Class: An abstract class cannot be instantiated directly and must be inherited. This class can contain abstract methods that subclasses must implement.
- Interface Class: An interface in Java is like a contract that classes must adhere to. The methods in an interface have no body, and the classes must provide the implementation for these methods.
Working with Eclipse
How to Create a Java Class in a Project Using Eclipse IDE
-
Create a New Java Project:
- Open Eclipse.
- Select File > New > Java Project.
- In the window that appears, enter the project name (e.g.,
MyFirstJavaProject
), then click Finish.
-
Create a New Class in the Project:
- After creating the project, in the Project Explorer on the left, find the project you just created.
- Right-click on the src folder (if you don’t have this folder, create a
src
folder). - Select New > Class.
- The New Java Class window will appear.
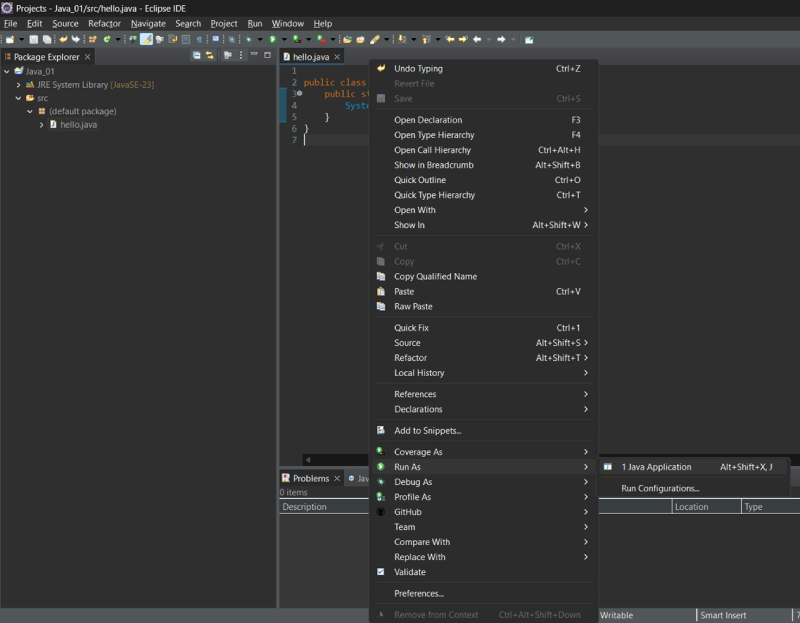
-
Enter Class Information:
- In the New Java Class window, you need to enter:
- Class Name: Example:
HelloWorld
. - Check public static void main(String[] args) if you want to automatically generate the
main
method (for simple Java programs). - Choose a Package (optional) if you want the class to be in a specific package (e.g.,
com.myapp
).
- Class Name: Example:
- In the New Java Class window, you need to enter:
-
Complete Class Creation:
- After entering the information, click Finish. The new class will be created in the
src
folder of the project and will open in the Eclipse working window.
- After entering the information, click Finish. The new class will be created in the
main
Method in a Class
Quickly Create the When creating a new class in Eclipse, you may want to quickly add a main
method to start the program. Instead of typing the entire syntax of the main
method, you can use a shortcut:
- In your class, type the keyword
main
. - Then press
Ctrl + Space
, Eclipse will show suggestions. - Select the first suggestion or press
Enter
to automatically complete themain
method.
Example:
When you type the keyword main
and press Ctrl + Space + Enter
, Eclipse will generate the following code:
public static void main(String[] args) {
// TODO Auto-generated method stub
}
Run a Program in Eclipse
To run a program in Eclipse, you can follow one of three methods:
- Method 1: Run from the code window: Open the Java file you want to run. Then right-click anywhere in the program window and select Run As > Java Application.
- Method 2: Run from the toolbar: After opening the program, click the Run button (green triangle icon) in the top-right corner of the Eclipse window to run the program.
- Method 3: Run from the menu bar: You can select Run > Run from the top menu bar.
Conclusion
A class in Java is a powerful and essential tool for organizing source code, helping you easily create and interact with objects. Understanding classes and their components is the first step towards building effective and maintainable Java programs.
As you continue learning Java programming, getting familiar with concepts like inheritance, polymorphism, and abstraction will help you use classes proficiently and develop more complex applications.
Next lesson: Lesson 4. How to Declare Variables in Java
Latest Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
Related Posts
Lesson 26. How to Use break, continue, and return in Java | Learn Java Basics
A guide on how to use break, continue, and return statements in Java to control loops and program execution flow effectively.
Lesson 25. The do-while Loop in Java | Learn Basic Java
A detailed guide on the do-while loop in Java, including syntax, usage, examples, and comparison with the while loop.
Lesson 24. How to Convert Decimal to Binary in Java | Learn Basic Java
A guide on how to convert numbers from the decimal system to the binary system in Java using different methods, with illustrative examples.
Lesson 23. How to Use the While Loop in Java | Learn Java Basics
Learn how to use the while loop in Java with syntax, real-world examples, and practical applications in Java programming.
